Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial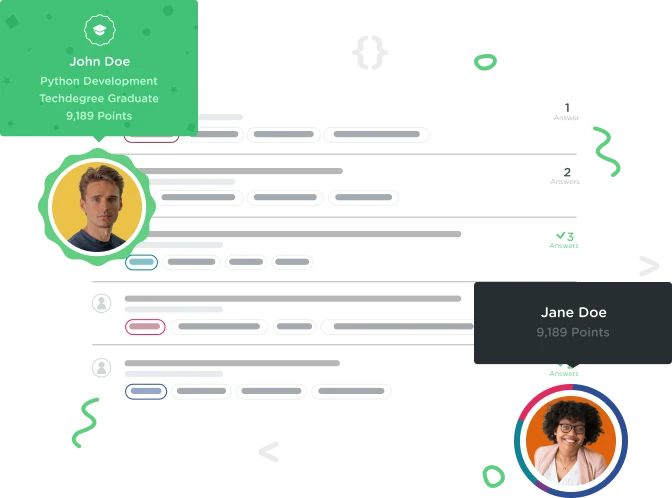

Marissa Smith
16,609 PointsClosures- console returning a function instead of executing code inside of inner function
function makeBirdCounter() {
let count=0;
return function (){
count += 1;
return count + ' birds';
}
}
console.log(makeBirdCounter());
this returns
Æ (){
count += 1;
return count + ' birds';
}
but in the video it does the calculation returning
1 birds
2 birds
3 birds
depending on how many times its ran..whats going on?
2 Answers

odzer
20,483 Points"Why do we have to pass the function into a variable and then call it, why can't you just call the function directly?"
You can't call it directly because calling it only returns the inner function, it does not execute the inner function. So if you console.log(makeBirdCounter()); you see the inner function in the console, you do not see the output of the inner function. But you can run it directly by doing console.log(makeBirdCounter()());
However, in this case, it will always log 1 bird, as the count variable inside the outer function will be set to 0 each time the outer function is called.
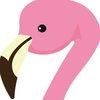
Dave StSomeWhere
19,870 PointsYou're missing the part in the video where he assigns the anonymous function returned from makeBirdCounter() to a variable and then executes the returned function. Hopefully you understand the overall concept of closures a bit better...
You just need to add the new variable, something like:
function makeBirdCounter() {
let count = 0;
return function () {
count += 1;
return count + ' birds';
}
}
//console.log(makeBirdCounter());
let functionVariable = makeBirdCounter();
console.log(functionVariable());
console.log(functionVariable());
console.log(functionVariable());
console.log(functionVariable());
// outputs
1 birds
2 birds
3 birds
4 birds

Marissa Smith
16,609 PointsWhy do you have to do that?
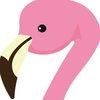
Dave StSomeWhere
19,870 PointsI'm not sure what you are asking. Could you elaborate on your question a little bit?

Marissa Smith
16,609 PointsWhy do we have to pass the function into a variable and then call it, why can't you just call the function directly?
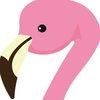
Dave StSomeWhere
19,870 PointsI think the real answer is that these function features is a useful way for the instructor to demonstrate the closure concept.
So, being able to assign the returned function to a variable, you can see (as the main purpose of the video) that each variable assigned this anonymous function has it own scope, containing the count variable. An this is what is referred to as a closure.
If that doesn't make sense, it is probably worth it to watch the video again, without getting hung up on the function stuff.
Functions have many great features. Yes, you can call functions directly, you can store functions in objects, along with many other spiffy features... Hopefully that helps a bit.