Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial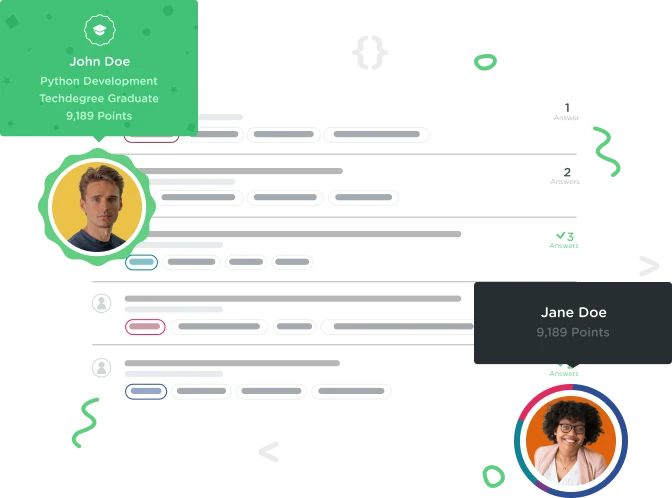

Nader Alharbi
2,253 PointsClasses and methods concept
I already passed the question but i was confused when i saw it is requested to define a method with two arguments.
If student is a class, shouldn't every student grade be different? that means it should be an attribute not just a variable. One common variable such as grades curve can be used for the methods.
class Student:
name = "Enter students name"
grade = "Enter students grade"
def praise(self):
return print("You inspire me, {}".format(self.name))
def reassurance(self):
return print("Chin up, {}. You'll get it next time!".format(self.name))
def feedback(self, grade_curve):
if self.grade + grade_curve > 50:
return self.praise()
if self.grade + grade_curve <= 50:
return self.reassurance()
nader = Student()
nader.name = "Nader"
nader.grade = 89
alex = Student()
alex.name = "alex"
alex.grade = 43
grade_curve = 4
nader.feedback(grade_curve)
alex.feedback(grade_curve)
Wouldn't this code make more sense than the challenge? I am still confused about classes concept
2 Answers
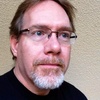
Chris Freeman
Treehouse Moderator 68,426 PointsWhile built-in objects such as int
, list
, set
, dict
, etc. can hold chucks of data, user created classes gain their power from being able to contain different related data, and provide specific functions (called methods) to manipulate the data.
One analogy is to think of a class in terms of English:
- a class could be a noun that represents an actual thing
- class attributes could be the adjectives that describe quality about the noun
- class methods are the verbs used to take action and modify the adjectives.
A class is something that might be stored in a database or spreadsheet where:
- each row is an instance of a class
- each column is an attribute of the instance
Lastly, you may have heard that everything in Python is an object. Well, all objects derive from some class. All the built-in standard type objects, such as str are classes, with many methods:
‘capitalize’, ‘casefold’, ‘center’, ‘count’, ‘encode’, ‘endswith’, ‘expandtabs’, ‘find’, ‘format’, ‘format_map’, ‘index’, ‘isalnum’, ‘isalpha’, ‘isdecimal’, ‘isdigit’, ‘isidentifier’, ‘islower’, ‘isnumeric’, ‘isprintable’, ‘isspace’, ‘istitle’, ‘isupper’, ‘join’, ‘ljust’, ‘lower’, ‘lstrip’, ‘maketrans’, ‘partition’, ‘replace’, ‘rfind’, ‘rindex’, ‘rjust’, ‘rpartition’, ‘rsplit’, ‘rstrip’, ‘split’, ‘splitlines’, ‘startswith’, ‘strip’, ‘swapcase’, ‘title’, ‘translate’, ‘upper’, ‘zfill’
As a class, getting the uppercase version of a string is as easy as calling the class method upper()
using the “dot” notation:
>>> s = 'Python is Fun!'
>>> s.upper()
‘PYTHON IS FUN!’
I encourage you to keep at it. The usefulness of classes will become more evident as you progress through the Treehouse coursework.
As always, post back if you need more help. Good luck!!!
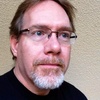
Chris Freeman
Treehouse Moderator 68,426 PointsMany of the challenge questions are minimized to get a coding concept tested. I see your point that needing to supply the grade
seems more work than storing the grade as an attribute.
Since students may have many grades, the usage model here may be to store all the grades in another Grades
class, then use the Student.feedback()
method as the way to generate the praise. It seems better to have the feedback
method part of the Student
class, than in the Grades
class.
I would be happy to explain class concepts to you. What part is still confusing?

Nader Alharbi
2,253 PointsFunctions, loops, if conditions and sets are powerful tools and i know where to apply them.
Classes on the other hand ... they seem ... just painful useless tools (i know they aren't but its just my feeling)
Also, my brother studies computer engineering and i asked him about classes and he has no clue about them (for the languages he learned)
Nader Alharbi
2,253 PointsNader Alharbi
2,253 PointsThat is well explained. Do you happen to know if i can access the class str for example and see the actual code?
Chris Freeman
Treehouse Moderator 68,426 PointsChris Freeman
Treehouse Moderator 68,426 PointsThe built-in types, and underlining structure of Python, are written in a version of C called Cython. The Cython code for the built-in functions can be found here. Warning: It's not easy reading!
You may get more mileage from looking at the Classes tutorial from the Python docs.