Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial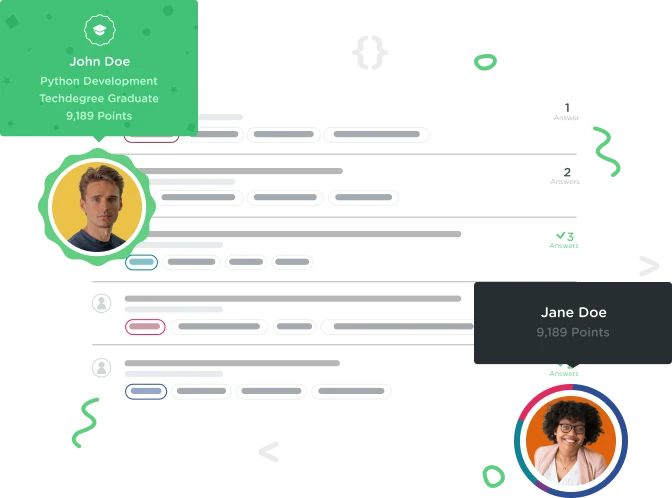

Andrew Bickham
1,461 Pointsclass RaceCar
I keep getting error: can't find class 'RaceCar', which I can't understand why, I believe everything to be correct
class RaceCar:
def __init__(self, color, fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
for key, value in kwargs.item():
setattr(self, key, value)
2 Answers

Darryl Mah
5,492 PointsOne of the really finicky things with python is indentation. It trips me up all the time. Your code is solid, with the exception of the indentation, (these little mistakes right? Lol) Since you want the “for loop” to be inside your init move that over so it becomes encompassed and you’re good too go!
Hope this helps!

Andrew Bickham
1,461 Pointsclass RaceCar:
def __init__(self, color, fuel_remaining, laps, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = 0
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
self.length = fuel_remaining - length * 0.125
self.laps += 1
i checked everything, made sure everything was where it needed to be but i keep getting a bummer message

Darryl Mah
5,492 PointsTried posting earlier with more detail but apparently I’m getting a 500 error. Lol don’t want to type out the explanation again. So here is just the answer.
class RaceCar:
def __init__(self, color, fuel_remaining, laps = 0 **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = laps
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
self.fuel_remaining = self.fuel_remaining - (length * 0.125)
self.laps += 1
[MOD: added ```python formating -cf]

Andrew Bickham
1,461 Pointsthanks again ill analyze your code before i move on

Dan B
6,155 PointsDarryl this is not a correct answer, even if it somehow manages to pass the challenge.
Laps should not be an argument being passed into init we are using **kwargs to pass laps in because we aren't setting a variable for laps directly under class.
Also self.color and self.fuel_remaining need to be directly part of the RaceCar class.
So like this:
class RaceCar:
color = 'blue'
fuel_remaining = 10
# We set the laps to 0 here because if we set it anywhere else all instances
# would start with how many laps happened last time
def __init__(self, color, fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = 0
for key, value in kwargs.items(): # Using kwargs here because we aren't
setattr(self, key, value) # defining laps in the class but we still need laps
def run_lap(self, length):
self.fuel_remaining -= length * 0.125
self.laps += 1
Andrew Bickham
1,461 PointsAndrew Bickham
1,461 Pointsso simple, thank you