Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial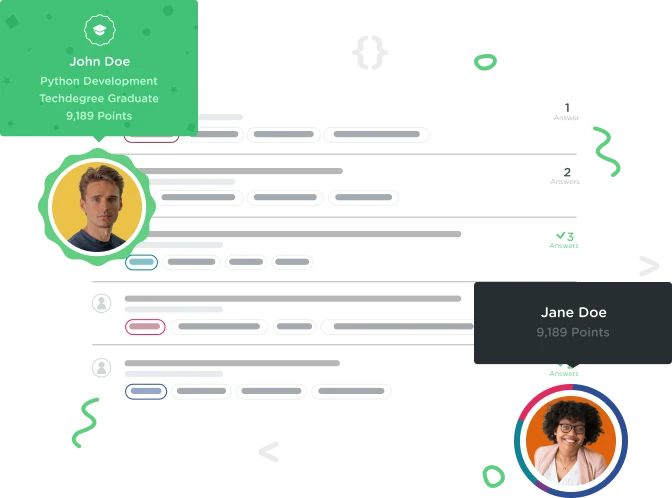

Zahra Alsuliman
Courses Plus Student 2,314 PointsChallenge Task 4 of 4 of slice section related to reverse_evens is not checking my work correctly. a[::-2] is my answer.
Challenge Task 4 of 4 of slice section related to reverse_evens is not checking my work correctly. a[::-2] is my answer.
def first_4(a):
return a[:4]
def first_and_last_4(a):
return a[:4]+a[-4::]
def odds(a):
return a[1::2]
def reverse_evens(a):
return a[::-2]
3 Answers

Mike Wagner
23,559 Pointsreverse_evens
will be a bit trickier than the others, as you will need to account for the list length and modify your starting position to account for lists with both odd and even lengths. This will need some form of an if-else
or a functional alternative. I would recommend playing with your code in an interpreter like repl.it so you can print out the results to a console and see what you're actually returning from the code.

Zahra Alsuliman
Courses Plus Student 2,314 PointsMike Wagner Thanks again, Mike, for taking the time to explain. I see your point. But Maybe my wording of the question was confusing. The question is to reverse the even indices if I understand the question correctly. So, the list is arbitrary. Here is the original question from the code challenge exercise on the topic of slices. "Make a function named reverse_evens that accepts a single iterable as an argument. Return every item in the iterable with an even index...in reverse. For example, with [1, 2, 3, 4, 5] as the input, the function would return [5, 3, 1]." Is my understanding of the question correct?

Mike Wagner
23,559 PointsZahra Alsuliman - The starting list defines the finished result in only one way. Let's look at this in a different way. Rather than the values, we'll look at the indices, since that's what the task statement asks for anyway.
"Make a function named reverse_evens that accepts a single iterable as an argument. Return every item in the iterable with an even index...in reverse. For example, with [1, 2, 3, 4, 5] as the input, the function would return [5, 3, 1]."
So, with an odd-length iterable, we have the indices
[0, 1, 2, 3, 4]
while for an even length we have
[0, 1, 2, 3, 4, 5]
Since the original question wants us to
"Return every item in the iterable with an even index...in reverse"
if we reverse these lists first we wind up with
[4, 3, 2, 1, 0] # obviously in indices 0-4 respectively
# and
[5, 4, 3, 2, 1, 0] # indices 0-5
Now it might be a little bit more clear that, by taking every even index of the now-reversed list,
[5. 3. 1] # remember, our values are the indices in our example
we're actually returning the original odd index values in an even-length list. This problem only presents itself with iterable that is even-lengthed, because (looking in our reversed list above) we can see that the odd-length iterable, when reversed, actually still has the original indices in even positions within the list.
This might be getting a bit winded, so I'll leave it with this final thought for now. Since the question specifically asks for the items at an even index, it's generally a better idea to build the new list by first taking the even index values and putting them in the new list. While you can reverse the list first, in doing so, you actually have to account for the len(starting_list)
to check if it is odd- or even-lengthed. If it is even, then you would simply start at position 1 instead of position 0 when you build the new_list
out of the reversed starting_list
.
I hope that makes sense.

Zahra Alsuliman
Courses Plus Student 2,314 PointsMike Wagner This is so much clear now. The question got me :) Thanks a lot for the explanation.

Mike Wagner
23,559 PointsZahra Alsuliman - of course. I'm glad to help :)
Zahra Alsuliman
Courses Plus Student 2,314 PointsZahra Alsuliman
Courses Plus Student 2,314 PointsThanks for your comment, Mike! So, here is what I found. I have tried my solution on the terminal, and it seems to be working fine. I tried both odd and even lengths. Now, here is the surprising thing. I went back to the question on the website, and I tried the following two solutions:
def reverse_evens(a): # (this one was marked wrong)
def reverse_evens(a): # (this one was marked right, finally :) )
Honestly, I don't have an explanation as to why the website is marking the first one wrong. The only thing I can think of is that the website checks my solution in a "literal" way against its predefined solution.
Mike Wagner
23,559 PointsMike Wagner
23,559 PointsZahra Alsuliman - The first one (that you said was marked wrong) is actually a correct in a scenario where the iterable you're testing against is of odd length, but only then. The second solution (that you said was marked right) is the correct solution if the iterable is both even and odd. Here's the basic rundown of why:
b = a[::-1] # Take everything from a, in reverse order
If we set a test up with something like
test_list = list(range(10))
, we get this as a result forb
:[9, 8, 7, 6, 5, 4, 3, 2, 1, 0]
however, if we set it as
test_list = list(range(9))
we get this:[8, 7, 6, 5, 4, 3, 2, 1, 0]
Now, when we run
b = b[::2] # take every entry at the second step
You might see how we're building our array and that in an odd-length list we get:
[8, 6, 4, 2, 0]
but with an even we get
[9, 7, 5, 3, 1]
This means we're actually only keeping the odd indices from the original even-length list.
Your second solution, and the correct one, given the setup you've created, doesn't care how long the list is. That's because we are doing our stepping first, which guarantees that we are only taking the even indices from
a[]
and plugging them into our new listb[]
indices.This means when we then reverse their order, all the values will be the same, regardless of whether the original list we received was of odd-length or of even-length. Hopefully, that makes things more clear. Sometimes you just have to toy around with these things yourself in an interpreter with a few
print()
calls scattered around to see what's going on and get a feel for things.