Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial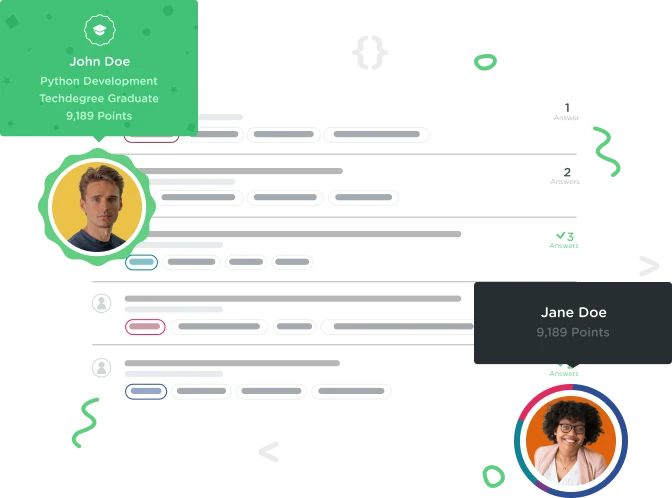

Chris Roper
11,001 PointsChallenge Task 1 of 1 Without changing the variables, create a SINGLE conditional statement around the echo command t
There's probably something obvious I'm not getting, but i'm not sure what it means by asking to check for username? I fine with checking if the role is not equal to admin, inside an if statement. Any help would be appreciated.
<?php
$username = 'sketchings';
//Available roles: author, editor, admin
$role = 'editor';
//add conditional statement
if($role != 'admin') {
echo "You do not have access to this page. Please contact your administratior.";
}
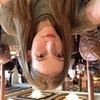
Kat Stacy
41,658 PointsYou can not use the pipe || (or) operator here because both of the values must be true for a user to gain access to the page. The must have a username and they must have an admin role. You need to make sure to use the ampersand && (and) operator.
//NOT like this
if($username || $role != 'admin')
//do it like this
if(&username && $role != 'admin')
Also, you need to check to see if the $username is set. You can do this two ways that I know of.
//Way 1
if($username == true && $role != 'admin')
//Way 2
if(isset($username) && $role != 'admin')
//Personally I prefer the second way as it is more descriptive and for me easier to read and understand.
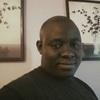
Tinashe Pride Mudzimu
5,886 Pointsif ($username = is_string($username) && $role != 'admin') { echo "You do not have access to this page. Please contact your administrator. " } ?>
8 Answers

Advaith Thachat
831 PointsHey I think I found a way out!
<?php
$username = 'sketchings';
//Available roles: author, editor, admin
$role = 'editor';
//add conditional statement
//the $username is evaluated to TRUE and
if ($username && $role != 'admin') {
echo "You do not have access to this page. Please contact your administratior.";
}
Hope this helps.. Happy Coding... :-)
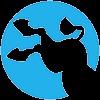
Leanne Millar
9,555 PointsThank Advaith. This helped a lot. However, the code above won't pass due to a typo. You've put an extra "i" in administrator.
<?php
$username = 'sketchings';
//Available roles: author, editor, admin
$role = 'editor';
//add conditional statement
//the $username is evaluated to TRUE and
if ($username && $role != 'admin') {
echo "You do not have access to this page. Please contact your administrator.";
}
?>
This code works fine
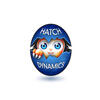
Zachary Scott
1,650 PointsThis worked for me:
<?php
$username = 'sketchings';
//Available roles: author, editor, admin
$role = 'editor';
//add conditional statement
if (is_string($username) && $role != 'admin') {
echo "You do not have access to this page. Please contact your administrator.";
}
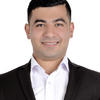
Samandar Mirzayev
11,834 Points<?php
$username = 'sketchings';
//Available roles: author, editor, admin
$role = 'editor';
//add conditional statement
if (isset ($username) && $role != 'admin'){
echo "You do not have access to this page. Please contact your administratior.";
}
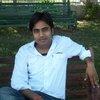
Vipin Singh
15,268 Points<?php
$username = 'sketchings';
//Available roles: author, editor, admin
$role = 'editor';
//add conditional statement
if ($username == true && $role != 'admin') {
echo "You do not have access to this page. Please contact your administratior.";
}
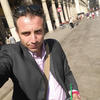
Andrew Lenti
Front End Web Development Techdegree Student 7,193 Points<?php $username = 'sketchings'; //Available roles: author, editor, admin $role = 'editor';
//add conditional statement if($role != 'admin' || is_string($username)) { echo "You do not have access to this page. Please contact your administratior."; }
?>
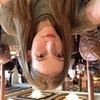
Kat Stacy
41,658 PointsIf you don't mind can you tell me why you use the || . I tried your code and it passes but I am not sure why. Don't both conditions have to be true for this task, so we should use the &&? I only ask because I am just curious because maybe you know something I don't know.

David Ruck
309 Points<?php
$username = 'sketchings';
//Available roles: author, editor, admin
$role = 'editor';
//add conditional statement
if (isset($username) && $role != 'admin') {
echo "You do not have access to this page. Please contact your administrator.";
}
// This is the correct code.

David Schreiber
Front End Web Development Techdegree Graduate 16,402 PointsThis worked for me:
if ($username != '' && $role != 'admin') { echo "You do not have access to this page. Please contact your administrator."; }

Chris Roper
11,001 PointsChris Roper
11,001 PointsNever mind I worked it out watching the next video.
if($username || $role != 'admin') { echo "You do not have access to this page. Please contact your administratior."; }