Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial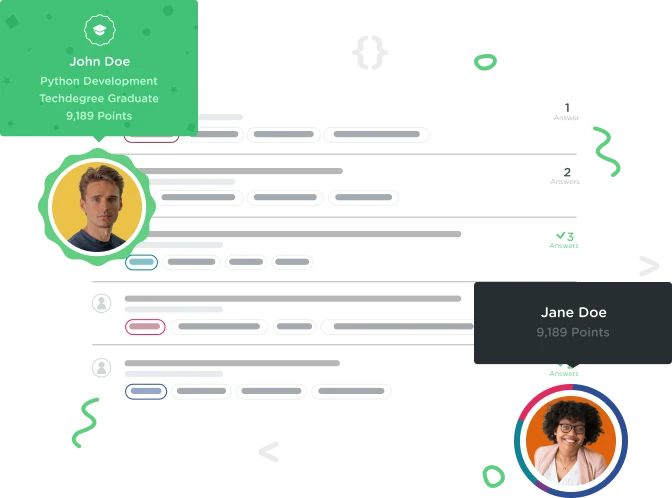

Kari Soto
1,836 PointsChallenge problem with average tongue length
Here is my code:
namespace Treehouse.CodeChallenges { class FrogStats { public static int GetAverageTongueLength(Frog[] frogs) { int final = 0; int amount = 0; for(int index = 0; index < frogs.Length; index++) { final = final + frogs[index]; amount = amount + 1; } final = final / amount; return final; } } }
Here is the idea:
this method would run until index == the amount of values in the array frogs. for(int index = 0; index < frogs.Length; index++) {...}
In that method, this command is run: final = final + frogs[index]; the value "final" is every value in the array added together.
After that, the value of "amount" would increase every time the "for" method is run. amount = amount + 1;
then, it would get the average and return it. final = final / amount; return final;
I don't see any reason why this would not work, but I get the exception:
FrogStats.cs(10,25): error CS0019: Operator +' cannot be applied to operands of type
int' and `Treehouse.CodeChallenges.Frog'
I only have a basic understanding of what it means. I believe that something is wrong with this part:
final = final + frogs[index];
but I am not sure what, it seems alright to me.
EDIT: sorry about the formatting. Apparently treehouse doesnt carry over some spaces. It may be a little hard to read
1 Answer

andren
28,558 PointsThe problem is that the frogs array is not an array of numbers, but an array of frog objects, so frogs[index] will result in a single frog object.
The frog's tongue length which is what you are trying to average is stored as a property on the frog object, so to access it you have to use dot notation like this: frogs[index].TongueLength
You also seem to have changed the return type of GetAverageTongueLength from double to int, which is wrong, the challenge will not pass unless the return type is set to double.
If you fix those two issues your code should run fine, though I would also like to add that the amount variable you create is not necessary as you can get the amount of frogs simply by using frogs.Length, like you do when you setup the for loop itself.
Kari Soto
1,836 PointsKari Soto
1,836 PointsThank you!