Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial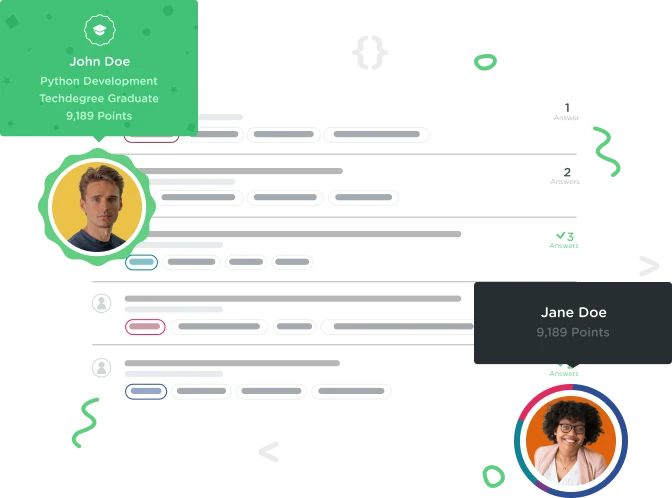

Vic Mercier
3,276 PointsChallenge firstLi
For example, notice when I click on the Up button of the top list item,
nothing happens, because they can't go up any further than the top, right?
So using first element, child, you could remove the Up button here.
So the app doesn't have a nonfunctional button.
I don't know how to do that.
2 Answers
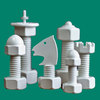
Steven Parker
241,970 PointsThat was covered in the Removing Nodes video.
You might want to review that one. But be aware that if you remove the "up" button from the top item (and I assume the "down" button from the bottom), you'll need to recreate them if those items are moved with the other button.
As an alternative enhancement, I would recommend just setting the button's "disabled" property, which will change the visual appearance in a way most users will recognize as meaning "not active". Then you can just clear that property when the item is moved.

Vic Mercier
3,276 PointsCan you show me your code

Vic Mercier
3,276 Pointsconst removeItemInput = document.querySelector("input.removeInput");
const toggleList = document.getElementById("togglelist");
const listDiv = document.querySelector(".list");
const listDivUl = document.querySelector("ul");
const myInput = document.querySelector("input.description");
const myP = document.querySelector("p.description");
const button = document.querySelector("button.description");
const addItemInput = document.querySelector("input.addItemInput");
const addItemButton = document.querySelector("button.addItemButton");
const removeButton = document.querySelector("button.removeItemButton");
const lis = listDivUl.children;
function attachListItemsButton(li){
//Up button
let up = document.createElement("button");
up.className = "up";
up.textContent = "up";
li.appendChild(up);
//Down button
let down = document.createElement("button");
down.className = "down";
down.textContent = "down";
li.appendChild(down);
//Remove button
let remove = document.createElement("button");
remove.className = "remove";
remove.textContent = "remove";
li.appendChild(remove);
}
listDivUl.addEventListener("click", (event) => {
if(event.target.tagName == "BUTTON"&& event.target.className == "remove"){
let li = event.target.parentNode;
let ul = li.parentNode;
ul.removeChild(li);
}
});
listDivUl.addEventListener("click", (event) => {
if(event.target.tagName == "BUTTON" && event.target.className == "up"){
let li = event.target.parentNode;
let previousLi = li.previousElementSibling;
let ul = li.parentNode;
if(previousLi ){
ul.insertBefore(li, previousLi);
}
}
});
listDivUl.addEventListener("click", (event) => {
if(event.target.tagName == "BUTTON" && event.target.className == "down"){
let li = event.target.parentNode;
let nextLi = li.nextElementSibling;
let ul = li.parentNode;
if(nextLi){
ul.insertBefore(nextLi, li);
}
}
});
toggleList.addEventListener("click", () =>{
if(listDiv.style.display == "none"){
toggleList.textContent = "Hide list";
listDiv.style.display = "block";
}else{
toggleList.textContent = "Show list";
listDiv.style.display = "none";
}
});
button.addEventListener("click", () => {
myP.textContent = myInput.value+" : " ;
myInput.value = "";
});
addItemButton.addEventListener("click", () => {
let ul = document.getElementsByTagName("ul")[0];
let li = document.createElement("li");
li.textContent = addItemInput.value;
let firstChild = ul.firstElementChild;
attachListItemsButton(li);
ul.appendChild(li);
let first = ul.firstElementChild;
let button = document.getElementsByClassName("up")[0];
first.removeChild(button);
addItemInput.value = "";
});
Is that better?
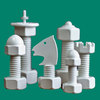
Steven Parker
241,970 PointsWhen posting code, always use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.
And here's the basic code for making a button disabled (and not):
var button = event.target;
button.disabled = true; // button stops working and appears gray
button.disabled = false; // button works again

Vic Mercier
3,276 PointsDo the button move?
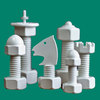
Steven Parker
241,970 PointsA disabled button won't move, but it will look different (gray color) and will no longer respond to clicks.
Steven Parker
241,970 PointsSteven Parker
241,970 PointsIt looks this is a triplicate of this other question, and also this question too.