Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial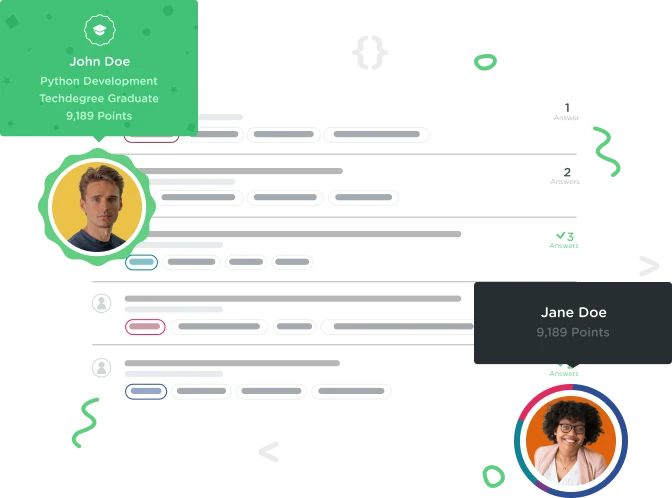

Jamar Slade
2,480 PointsCan't get my Item count message to print
I'm not sure if I've been starring at my screen for entirely too long or I'm just missing something here. Either I can't seem to get my item count to print after every item i type in. It seems once i click done, it prints the message but it only has one item regardless of how man I actually input. My code is below.
Create a new empty list named shopping list
shopping_list = []
Create a function named add_to_list that declares a parameter named item
# add the item to the list
def add_to_list(item): shopping_list.append(item)
notify the user that the item was added, state the number of items on list currently
print("Item was added to the list! There are currently {} items on the list".format(len(shopping_list)))
def show_help(): print("What should we pick up at the store? ") print(""" Enter 'DONE' to stop adding items Enter 'HELP' to for this help""")
show_help() while True: new_item = input("> ")
if new_item == "DONE":
break
elif new_item == "HELP":
show_help()
continue
Call add_to_list with new _item as an argument
add_to_list(new_item)
2 Answers

Lukasz Gorski
1,821 PointsHi Jamar,
Basically I can see two things that could be modified:
You can print within loop, so you will see number of products on the list:
Please remember to indent code within loop and if/elif statements.
while True:
print("Item was added to the list! There are currently {} items on the list".format(len(shopping_list)))
new_item = input("> ")
if new_item == "DONE":
break
elif new_item == "HELP":
show_help()
add_to_list(new_item)
continue
And to see at the end the results (whole shopping list) you can print out the results
print(shopping_list)

Jamar Slade
2,480 PointsThanks for the advice, adding to the loop fixed it. I'm not sure why they tried to get us to do it outside the loop. Thanks for your help
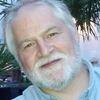
Jeff Muday
Treehouse Moderator 28,727 PointsThis might not be what you were looking for, but might be helpful.
Hint... you might want to research the term "global" in Python. This changes the scope of a variable (shopping_list) so you can reliably modify it with the add_to_list() function. Because list objects are "mutable" you don't actually need to include the "global" keyword, but it is still a good practice. In the example of add_to_total() the global is REQUIRED to modify total because it is a floating-point number.
https://www.geeksforgeeks.org/global-keyword-in-python/
see below:
shopping_list = []
total = 0
def add_to_list(item):
global shopping_list # although global is not required with a list, it is a good practice
shopping_list.append(item)
print("Item was added to the list! There are currently {} items on the list".format(len(shopping_list)))
def add_to_total(val):
global total # global is REQUIRED to access and modify the global variable "total."
total += val
add_to_list('milk')
add_to_total(3.99)
add_to_list('bread')
add_to_total(2.55)
print("Your shopping list: ")
print(shopping_list)
print("Your total costs:")
print(total)
Steven Parker
242,015 PointsSteven Parker
242,015 PointsUse the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.