Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial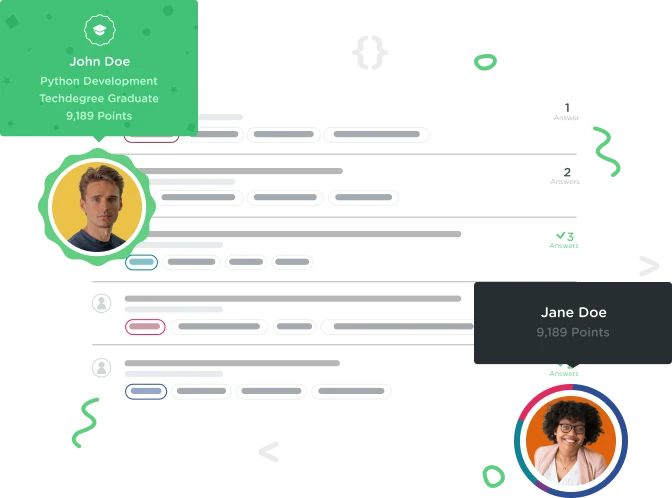

johnathanmyers
19,994 PointsCan't get both expressions to work at once in a multiline but they work individually.
Here is the goal...
"Create a new variable named contacts that is an re.search() where the pattern catches the email address and phone number from string. Name the email pattern email and the phone number pattern phone. The comma and spaces * should not* be part of the groups."
This is my code
import re
string = '''Love, Kenneth, kenneth+challenge@teamtreehouse.com, 555-555-5555, @kennethlove
Chalkley, Andrew, andrew@teamtreehouse.co.uk, 555-555-5556, @chalkers
McFarland, Dave, dave.mcfarland@teamtreehouse.com, 555-555-5557, @davemcfarland
Kesten, Joy, joy@teamtreehouse.com, 555-555-5558, @joykesten'''
contacts = re.search(r'''
^(?P<email>[-\w\d.+?]+@[-\w\d.]+)
(?P<phone>\d{3}[-\.\s]\d{3}[-\.\s]??\d{4})$
''', string, re.X | re.M)
I tried to break each line down in my pycharm ide to make sure they work independently and they do...
When I print the phone number line in my pycharm ide...
print(re.search(r'''
(?P<phone>\d{3}[-\.\s]\d{3}[-\.\s]??\d{4})
''', string, re.X | re.M))
I get this as the output...
_sre.SRE_Match object; span=(52, 64), match='555-555-5555'
And when I print the email line in the pycharm ide...
print(re.search(r'''
(?P<email>[-\w\d.+?]+@[-\w\d.]+)
''', string, re.X | re.M))
I get this as the output...
_sre.SRE_Match object; span=(15, 50), match='kenneth+challenge@teamtreehouse.com'
But when I try to print both...
print(re.search(r'''
^(?P<email>[-\w\d.+?]+@[-\w\d.]+)
(?P<phone>\d{3}[-\.\s]\d{3}[-\.\s]??\d{4})$
''', string, re.X | re.M))
I get "None" as the output.. Can't seem to get them to work together
Not sure why that is. I get the feeling it's a simple mistake I'm overlooking and if it where a snake it would be right in front of my face ready to bite me... A Python maybe? lol

johnathanmyers
19,994 PointsHey Adam,
Lines 2 and 3 of your code need to be indented correctly. Just add 4 spaces to the beginning of them.. Also, notice between the email and phone number there is a comma and a space in the string to match. That is what I had a problem with. just add an.. ,\s on it's own line between the two
(?P<email>[-\w\d.+]+@[-\w\d.]+)
,\s
(?P<phone>\d{3}-\d{3}-\d{4})
I highly suggest trying out pythex.org
4 Answers
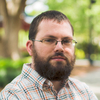
Kenneth Love
Treehouse Guest TeacherYour problem is actually fairly subtle. Your pattern uses ^
and $
which denote the beginning and of end of strings/lines, respectively. But look at the string we're matching. Are you patterns actually at the beginning or end of the string/lines? If they're not (hint: they're not), you don't need those two symbols.

johnathanmyers
19,994 PointsThanks Kenneth! I went back and watched the videos again. I ended up dropping the use of re.M and I added ,\s on a new line between the two. There's a website called https://regex101.com/#python that really helped a lot too because it visually shows you what you are matching as you are creating your code. Now, Moving forward.
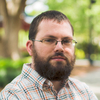
Kenneth Love
Treehouse Guest TeacherAnother great site for testing regex is pythex.org

johnathanmyers
19,994 PointsAwesome! Love the simplicity of the page layout. I'm collecting a nice library of tools now. Coming from a music background I know that tools don't make you a better musician, but they definitely improve the quality of your sound. Seems to apply to coding as well. Thanks again for your help.

Raymond Wach
7,961 PointsI haven't watched videos for this exercise so I may be missing some context, but I believe the issue is in the use of triple quotes. Specifically, triple quote string literals will include linebreaks and whitespace as is; this means that the literal pattern:
r'''
^(?P<email>[-\w\d.+?]+@[-\w\d.]+)
(?P<phone>\d{3}[-\.\s]\d{3}[-\.\s]??\d{4})$
'''
is actually
re'\n ^(?P<email>[-\\w\\d.+?]+@[-\\w\\d.]+)\n (?P<phone>\\d{3}[-\\.\\s]\\d{3}[-\\.\\s]??\\d{4})$\n'
I don't believe that will match anything in string
, which is why you get the result of None
.
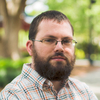
Kenneth Love
Treehouse Guest TeacherWhen you use re.X
(or re.VERBOSE
), the regex engine ignores all whitespace that isn't in a set.
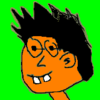
michaelangelo owildeberry
18,173 Pointsunbalanced parenthesis... (?P<email>[-\w\d.+]+@[-\w\d.]+)\t # email (?P<phone>(?\d{3})?-?\s?\d){3}-\d{4})\t # phone ... is it possible to do without any parenthesis?
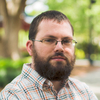
Kenneth Love
Treehouse Guest TeacherNo, you can't do groups or named groups without parentheses.
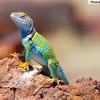
Adam Oliver
8,214 PointsThanks Johnathan the ,\s on a seperate line betwen fixed my code. Though, It still worked without indenting. Is the indenting just to make it look cleaner?

johnathanmyers
19,994 PointsYes, it's part of the best practices (Also known as PEP 8). Check out the link below.
https://www.python.org/dev/peps/pep-0008/#tabs-or-spaces
From what I've learned, as long as they both have the same indentation they will work. So in this case no spaces. I use 4 spaces just because that's what I was taught as the best practices. It just makes it easier if another programmer needs to work on your code... Or vice versa. :)
Adam Oliver
8,214 PointsAdam Oliver
8,214 PointsI don't understand why I keep getting 'None' back?