Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial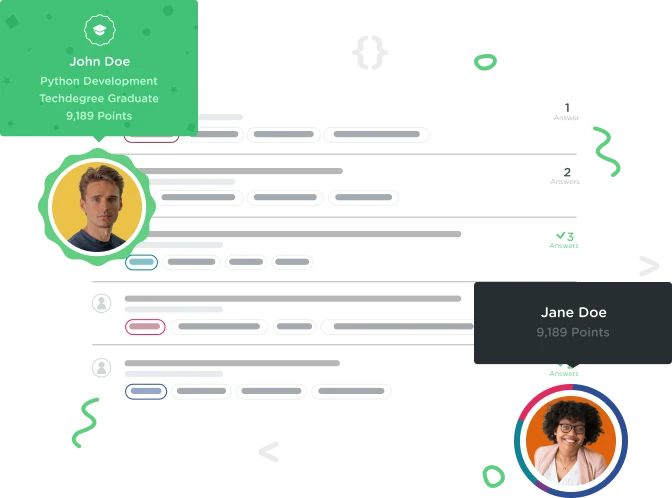

Adam Teale
10,989 PointsCan't compare `Song`s to `int`s
Hey guys,
As far as I can tell this should be working ok - when I test it locally it passes the comparisons. What am I missing?
Thanks!
Adam
class Song:
def __init__(self, artist, title, length):
self.artist = artist
self.title = title
self.length = length
def __eq__(self, other):
return int(self.length) == int(other.length)
def __gt__(self, other):
return int(self.length) > int(other.length)
def __lt__(self, other):
return int(self.length) < int(other.length)
def __le__(self, other):
return int(self.length) < int(other.length) or int(self.length) == int(other.length)
def __ge__(self, other):
return int(self.length) > int(other.length) or int(self.length) == int(other.length)
def __int__(self):
return int(self.length)
def __len__(self):
return int(self.length)

Adam Teale
10,989 PointsThank you Igorevi!

kgoldthorpe
7,802 PointsI have found this challenge to be very fickle. This worked for me, and it only worked for me when I copied it from someone else's answer in this forum. I typed this in verbatim and it didn't work, but when I copied and pasted the code, it worked.
def __int__(self):
return self.length
def __eq__(self, other):
return int(self) == other
def __lt__(self, other):
return int(self) < other
def __gt__(self, other):
return int(self) > other
def __le__(self, other):
return int(self) < other or int(self) == other
def __ge__(self, other):
return int(self) > other or int(self) == other
1 Answer

Neil Brown
22,514 PointsFor anyone else who has this problem.
It appears that what Kenneth is testing our code for is twofold: whether he can compare two songs together using the operators, and whether he can compare a song with an int using the operators. The code posted above will compare two songs with no error. But, if an integer is passed into the method, this code is calling other.length
, which is fine for a song object, but an integer does not have that attribute.
Each method needs to check if the parameter it is receiving is an integer. If it is, compare it to the parameter directly i.e int(self.length) > other
. If it is a song object then compare with the object's length. int(self.length) > other.length
Igor Ević
5,827 PointsIgor Ević
5,827 PointsYou can compare instance of Song class only with objects that have attribute length, because you use other.length in methods for comparisons.