Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial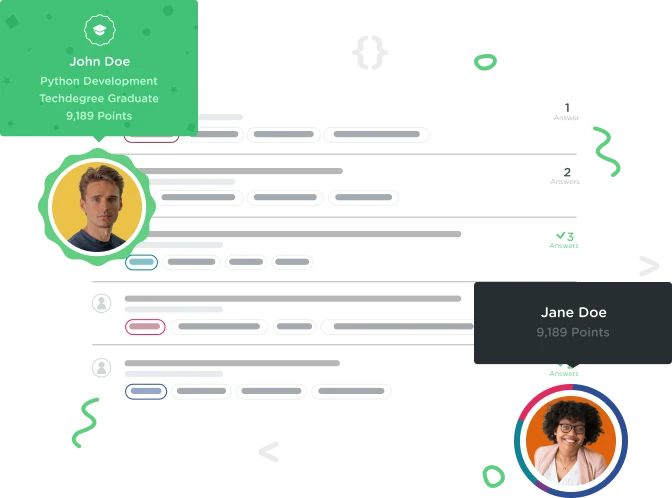
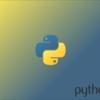
Kevin Faust
15,353 PointsCan someone read my code? im not sure why console says undefined
function print(message) {
document.write(message);
}
//all the questions and answers
var questions = [
["What is 50/2?", "25"]
["What OS has a green robot?", "ANDROID"]
["What is the capital of South Korea?", "SEOUL"]
]
//all the variables
var response="";
var answer="";
var numberRight=0;
var correct=[];
var wrong=[];
for (var i=0; i<questions.length; i++) {
response=prompt[i][0];
answer=[i][1];
if (response.toUpperCase()===answer) {
numberRight += 1;
correct.push(questions[i][0]);
}
else {
wrong.push(questions[i][0]);
}
}
//now correct has an array of the correct questions and wrong has an array of wrong questions
//create an ordered list of each array
for (var i =0; i<correct.length;i++) {
var completeCorrect = "<ol>";
completeCorrect += "<li>" + correct[i] +"<li>";
completeCorrect += "</ol>";
}
for (var i =0; i<wrong.length;i++) {
var completeWrong = "<ol>";
completeWrong += "<li>" + wrong[i] +"<li>";
completeWrong += "</ol>";
}
//display how many user got right
print(numberRight);
//display the correct and wrong
print("You got these questions correct: "+ completeCorrect );
print("You got these questions correct: "+ completeWrong);
The error that javascript is giving me is that: Uncaught TypeError: Cannot read property 'SEOUL' of undefined. but my SEOUL is in quotes so im not sure why it's saying it's undefined. also im not sure if i got my ending part right. i want to take everything pushed into the 2 arrays (correct and wrong) and organize them into another variable as an ordered list (completeCorrect and completeWrong). i also think i can use a function somewhere but i cant seem to figure out where.
Any help would be greatly appreciated. Cheers
4 Answers

Philip G
14,601 PointsYou declared the array indexes without refering to the array. This should finally work:
function print(message) {
document.write(message);
}
//all the questions and answers
var questions = [
["What is 50/2?", "25"],
["What OS has a green robot?", "ANDROID"],
["What is the capital of South Korea?", "SEOUL"]
]
//all the variables
var response="";
var answer="";
var numberRight=0;
var correct=[];
var wrong=[];
for (var i=0; i<questions.length; i++) {
response=prompt(questions[i][0]);
answer=questions[i][1];
if (response.toUpperCase()===answer) {
numberRight += 1;
correct.push(questions[i][0]);
}
else {
wrong.push(questions[i][0]);
}
}
//now correct has an array of the correct questions and wrong has an array of wrong questions
//create an ordered list of each array
for (var i =0; i<correct.length;i++) {
var completeCorrect = "<ol>";
completeCorrect += "<li>" + correct[i] +"<li>";
completeCorrect += "</ol>";
}
for (var i =0; i<wrong.length;i++) {
var completeWrong = "<ol>";
completeWrong += "<li>" + wrong[i] +"<li>";
completeWrong += "</ol>";
}
//display how many user got right
print(numberRight);
//display the correct and wrong
print("You got these questions correct: "+ completeCorrect );
print("You got these questions correct: "+ completeWrong);
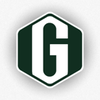
Gabor Salacz
9,020 Pointsfunction print(message) {
document.write(message);
}
//all the questions and answers
var questions = [
["What is 50/2?", "25"],
["What OS has a green robot?", "ANDROID"],
["What is the capital of South Korea?", "SEOUL"]
];
//all the variables
var response="";
var answer="";
var numberRight=0;
var correct=[];
var wrong=[];
for (var i=0; i<questions.length; i++) {
response=prompt(questions[i][0]);
answer=questions[i][1];
if (response.toUpperCase()===answer) {
numberRight += 1;
correct.push(questions[i][0]);
}
else {
wrong.push(questions[i][0]);
}
}
//now correct has an array of the correct questions and wrong has an array of wrong questions
//create an ordered list of each array
var completeCorrect = "<ol>";
for (var i =0; i<correct.length;i++) {
completeCorrect += "<li>" + correct[i] +"</li>";
}
completeCorrect += "</ol>";
var completeWrong = "<ol>";
for (var i =0; i<wrong.length;i++) {
completeWrong += "<li>" + wrong[i] +"</li>";
}
completeWrong += "</ol>";
//display how many user got right
print("Total CORRECT answers: " + numberRight + "<br><br>");
//display the correct and wrong
print("You got these questions correct: "+ completeCorrect );
print("You got these questions incorrect: "+ completeWrong);
There were multiple issues in the code:
- missing commas between the arrays inside the questions array,
- the prompt didn't use the questions array at all (the same mistake when assigning value to the answer variable),
- when creating the completeCorrect and completeWrong strings, the starting and ending <ol> tags must be added once (so you must take them out of the loop),
- when printing the numberRight value, there were no space / linebreak between it and the next text that gets printed after.
Hopefully this is what you were going after :) Cheers and keep up the good work;
Gabor.

Philip G
14,601 PointsYou have to separate the questions array with commas. This code should work:
function print(message) {
document.write(message);
}
//all the questions and answers
var questions = [
["What is 50/2?", "25"],
["What OS has a green robot?", "ANDROID"],
["What is the capital of South Korea?", "SEOUL"]
]
//all the variables
var response="";
var answer="";
var numberRight=0;
var correct=[];
var wrong=[];
for (var i=0; i<questions.length; i++) {
response=prompt[i][0];
answer=[i][1];
if (response.toUpperCase()===answer) {
numberRight += 1;
correct.push(questions[i][0]);
}
else {
wrong.push(questions[i][0]);
}
}
//now correct has an array of the correct questions and wrong has an array of wrong questions
//create an ordered list of each array
for (var i =0; i<correct.length;i++) {
var completeCorrect = "<ol>";
completeCorrect += "<li>" + correct[i] +"<li>";
completeCorrect += "</ol>";
}
for (var i =0; i<wrong.length;i++) {
var completeWrong = "<ol>";
completeWrong += "<li>" + wrong[i] +"<li>";
completeWrong += "</ol>";
}
//display how many user got right
print(numberRight);
//display the correct and wrong
print("You got these questions correct: "+ completeCorrect );
print("You got these questions correct: "+ completeWrong);
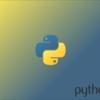
Kevin Faust
15,353 PointsHey Philip. Thanks for the reply. However now it's showing a new error: Uncaught TypeError: Cannot read property '0' of undefined
I don't really understand what this means