Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial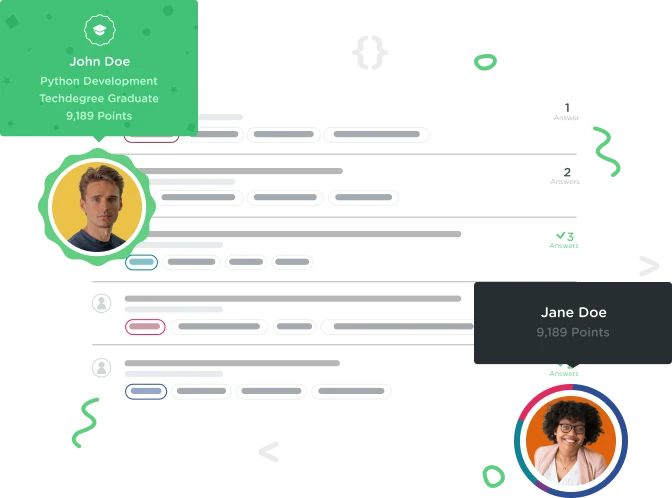

Kiran Matthews
963 PointsCan Someone Please help me!??
Hoe should i use the 'equals' method if i want to check if condition between two variable?
// I have imported a java.io.Console for you, it is named console.
String firstExample = "hello";
if(firstExample.equals("secondExample")){
console.printf("first is equal to second");
System.exit(0);
}
String secondExample = "hello";
String thirdExample = "HELLO";
3 Answers

Daniel Vigil
26,473 PointsKiran
The challenge is asking for you to compare two variables but looking at your code you are comparing the variable firstExample to the the string "secondExample" and not the variable. When using the variable name, you do not need the "".
You can read up on the equals method in the String class here: https://docs.oracle.com/javase/7/docs/api/java/lang/String.html#equals(java.lang.Object)

andren
28,558 PointsYour code is close to correct but there are some notable issues:
When you reference variables you should not enclose their names in quotes, quote marks are only used when you want to create a string, for any other type of data you should not use them.
You should not use the
System.exit()
command when doing these challenges. it's not really necessary since the program will exit once it's done running your code anyway, and it will cause issues with the code checker that verifies your code since it causes it to exit earlier than it is supposed to.You cannot reference a variable before it is declared in a method, in your solution you have the comparison code above the code that declares the second and third variable. It needs to be moved below them.
If you fix all of those issues you end up with this code:
String firstExample = "hello";
String secondExample = "hello";
String thirdExample = "HELLO";
if(firstExample.equals(secondExample)){
console.printf("first is equal to second");
}
Which will pass the first task.
Also do note that for challenges which have multiple parts like this one you have to keep your code from previous tasks around, so when you are completing task 2 do not change or remove the code you wrote in this task. If you do you won't be able to complete the challenge.

Kiran Matthews
963 PointsThank you so much!! This solved my issue. :)

Jana McKinnon
4,219 Pointsyou use == (two equal signs) to check two values against each other. A single equal sign, = , is the assignment operator, aka it assigns values to objects. Note that == may not always be used depending on how the object was created, same with the assignment operator. Some times you must override the operator. That's further down the line of your education path. You'll learn all about shallow and deep copy too :)

Jana McKinnon
4,219 Pointsalso in java there is the equals() and compareTo() functionswhich require Comparable to implemented.
Kiran Matthews
963 PointsKiran Matthews
963 PointsHey Daniel.. i tried removing the "". But for some reason I'am still getting an error.
Daniel Vigil
26,473 PointsDaniel Vigil
26,473 PointsKiran
Make sure the if statement falls under the variable assignments. As it is, it is looking for the variable secondExample prior to it even being declared. Also the System.exit(0) statement is not necessary.