Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial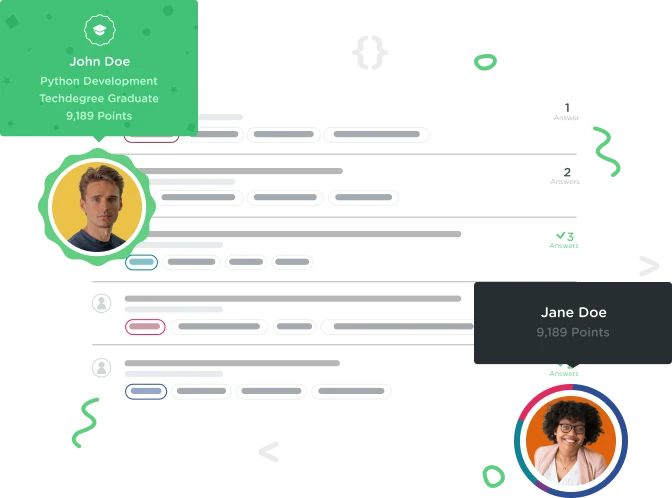

Daniel Hunter
4,622 Pointscan someone give me some pointers on normalisation on strings?
I am struggling to work out how to only allow letters or specific symbols to be passed in by the user. i tried converting the sting to a char array to call the Character.isLetter method on the char array but can't get it to work (see the commented code I moved to the bottom). please help.
Dan
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
private String normalizeDiscountCode(String discountCode){
if (! Character.isLetter(discountCode)){
throw new IllegalArgumentException("Invalid discount code.");
}
discountCode = discountCode.toUpperCase();
return discountCode;
}
}
/*for (char discountCodeCharacters = discountCode.toCharArray()){
if (! Character.isLetter(discountCodeCharacters)|| == '$'){
throw new IllegalArgumentException("Invalid discount code.");
}
}*/ // 1st attempt
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
2 Answers

Yanuar Prakoso
15,196 PointsHi Daniel
First of all you need to examine each character in the discountCode thus since discountCode is a String you need to convert it first to an array of characters. After that you can use for loops to iterate each character in the array of characters and validate its content.
Now for the IF statement. The validation said that IF the characters is NOT (a letter OR '$') the THROW something right? Therefor the IF statement should sound like this:
**IF (NOT(character is a Letter || charcater is 'S')) --> PS: character in Java uses single quotes (' ') while Strings uses double quotes (" ") and || means OR.
please note another thing Character.isLetter(character to be tested) is a method for Character (char) type object not String type object. Therefor you cannot just insert discountCode to the method like you did in your code. You need to test it using the character from the array list created from discountCode.
Here is my code if you want some reference:
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
private String normalizeDiscountCode(String discountCode){
char[] letters = discountCode.toCharArray(); //<--change it to array of characters first
for (char letter : letters){//<--for loop each character in the array of characters
if (! (Character.isLetter(letter) || letter == '$')){//<--the correct IF statement
throw new IllegalArgumentException("Invalid discount code.");
}
}
discountCode = discountCode.toUpperCase();
return discountCode;
}
I hope you can understand by reading the code. I hope this can help.

Daniel Hunter
4,622 PointsHi Yanuar,
Thanks that helped a lot, I knew I was getting close with my first attempt but I just couldn't get it to work. thank you.