Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial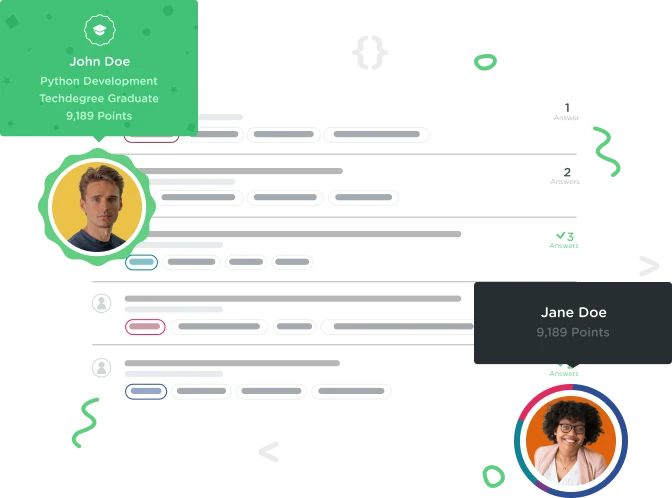

Vikram Pal
2,832 PointsCan someone explain the math being done in this problem to me? Is it picking a random number between lower and upper?
function getRandomNumber(lower, upper) { if ( isNaN(lower) || isNan(upper) ) { throw Error('Both arguments must be numbers.'); } return Math.floor(Math.random() * (upper - lower + 1)) + lower; }
1 Answer
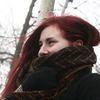
Ella Ruokokoski
20,881 PointsReturns a random integer between min (included) and max (included).
function getRandomIntInclusive(min, max) {
return Math.floor(Math.random() * (max - min + 1)) + min;
}
the + 1 is for the max value to be included too. If you omit the + 1 the max will be excluded. Math.random() returns a floating point number between 0 and 1 (1 is excluded) then that floating point number is multiplied by the number of numbers between the two points. Then to get a number between the two points you will add your minimum point to the result. Math.floor will return the number as an integer that is less or equal to the result.
demonstrating with numbers for clarity. You want a random number between 10 and 20 where the number 20 is included.
Math.floor(
Math.random() * (20 - 10 + 1) + 10
)
so if we say Math.random() would return for example 0.34
let random = 0.34 * 11 // 11 is the number of numbers between 10 and 20(included). this will return 3.74
random = random + 10 // we add the min value to get a number between 10 and 20 (20 included). and we get 13.74
console.log(Math.floor(random)) // returns 13
Vikram Pal
2,832 PointsVikram Pal
2,832 PointsThank you so much for explaining this! That really helped me a lot!