Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial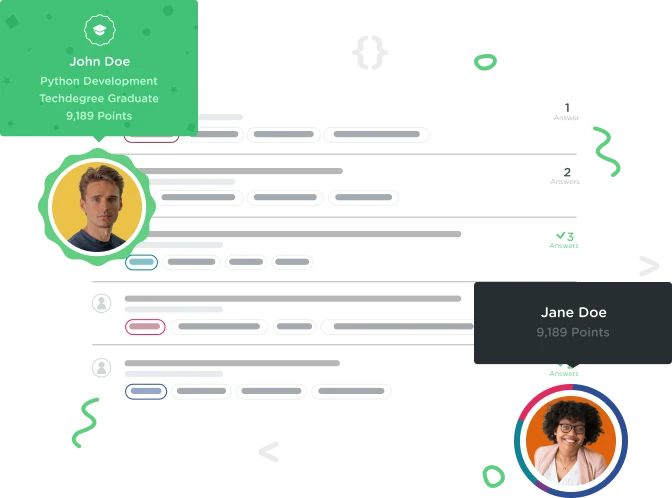

Peng Liu
Courses Plus Student 14,273 Pointscan not pass the teamwork TODO challenge
I have been around this challenge for a long time, just do not know why? when I run the main.java file, it says it can not load the censored words. It gives me error something related to matcher.java, I have changed the value passed into matcher.group(1) to match.group(0) so I can use trim() inside promptForWord(String phrase) method in Prompter.java when I test to see if it contains censored words; I also changed from 0 back to 1, and rid of the trim() chained after toLowerCase() inside promptForWord(String phrase) method as well, so far it does not work. Any advice? all my respected seniors and fellows...
Thanks Peng
package com.teamtreehouse;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Main {
public static void main(String[] args) {
// private BufferedReader mReader;
// mReader = new BufferedReader(new InputStreamReader(System.in));
Prompter prompter = new Prompter();
String story = null;
Template tmpl = new Template(story);
try {
// story = mReader.readLine();
story = prompter.promptForStory();
} catch (IOException ioe) {
ioe.printStackTrace();
ioe.getMessage();
}
}
}
package com.teamtreehouse;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
System.out.println(tmpl.render(results));
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
/**
* Prompts the user for the answer to the fill in the blank. Value is guaranteed to be not in the censored words list.
*
* @param phrase The word that the user should be prompted. eg: adjective, proper noun, name
* @return What the user responded
*/
public String promptForWord(String phrase) throws IOException {
mReader = new BufferedReader(new InputStreamReader(System.in));
System.out.printf("Please enter a %s", phrase);
String enteredWord = mReader.readLine();
while (mCensoredWords.contains(enteredWord.toLowerCase().trim())) {
System.out.printf("The %s is not appropriate, try again.", enteredWord);
enteredWord = mReader.readLine();
}
return enteredWord;
}
public String promptForStory() throws IOException {
// Prompter prompter = new Prompter();
// String story = null;
// Template tmpl = new Template(story);
// mReader = new BufferedReader(new InputStreamReader(System.in));
return mReader.readLine();
}
}
# This is essentially what I am testing
1. The user is prompted for a new string template (the one with the double underscores in it).
a. The prompter class has a new method that prompts for the story template, and that method is called.
2. The user is then prompted for each word that has been double underscored.
a. The answer is checked to see if it is contained in the censored words.
User is continually prompted until they enter a valid word
3. The user is presented with the completed story

Peng Liu
Courses Plus Student 14,273 Pointspackage com.teamtreehouse;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
Prompter prompter = new Prompter();
String story = null;
try {
story = prompter.promptForStory();
} catch (IOException e) {
e.printStackTrace();
}
}
}
This is my updated Prompter.java file:
package com.teamtreehouse;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
System.out.println(tmpl.render(results));
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
/**
* Prompts the user for the answer to the fill in the blank. Value is guaranteed to be not in the censored words list.
*
* @param phrase The word that the user should be prompted. eg: adjective, proper noun, name
* @return What the user responded
*/
public String promptForWord(String phrase) throws IOException {
System.out.printf("Please enter a %s", phrase);
String enteredWord = mReader.readLine();
String editedWord = enteredWord.toLowerCase();
while (mCensoredWords.contains(editedWord)) {
System.out.printf("The %s is not appropriate, try again.", enteredWord);
System.out.printf("Please enter a %s", phrase);
enteredWord = mReader.readLine();
editedWord = enteredWord.toLowerCase();
}
return enteredWord;
}
public String promptForStory() throws IOException {
System.out.println("Please tell us story about yourself:");
Prompter prompter = new Prompter();
String story = mReader.readLine();
Template tmpl = new Template(story);
prompter.run(tmpl);
return story;
}
}
When I debug or run the main.java file in intelliJ:
Couldn't load censored words java.nio.file.NoSuchFileException: resources/censored_words.txt Exception in thread "main" java.lang.NullPointerException at com.teamtreehouse.Prompter.loadCensoredWords(Prompter.java:34) at com.teamtreehouse.Prompter.<init>(Prompter.java:21) at com.teamtreehouse.Main.main(Main.java:10)
When I check work after copy past both Main.java and Prompter.java into challenge page, it gives me this:
Bummer! java.lang.NullPointerException(Look around Prompter.java line 79)
this is line 79:
String editedWord = enteredWord.toLowerCase();
I doubt it is the real problem, Since after debug, it says couldn't load censored words, this is what draws my attention:
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
Is this line right? I am new to java, sorry for my lack of knowledge:
Path file = Paths.get("resources", "censored_words.txt");
in order to point to censored_words.txt from Prompter.java file, we need to go: Prompter -> com.teamtreehouse -> src -> resources -> censored_words.txt. That is two levels up, parallel to resources, then down one level to censored_words.txt. Will
Path file = Paths.get("resources", "censored_words.txt");
do the trick?
Oh, after I check work, output.html shows:
Please tell us story about yourself:
Please enter a first promptjava.lang.NullPointerException
at com.teamtreehouse.Prompter.promptForWord(Prompter.java:79)
at com.teamtreehouse.Prompter.promptForWords(Prompter.java:62)
at com.teamtreehouse.Prompter.run(Prompter.java:40)
at com.teamtreehouse.Prompter.promptForStory(Prompter.java:96)
at com.teamtreehouse.Main.main(Main.java:9)
at JavaTester.run(JavaTester.java:77)
at JavaTester.main(JavaTester.java:39)
any advice? I am confused still...

Peng Liu
Courses Plus Student 14,273 PointsHi, everyone. this is my most recent updated code:
main.java file:
package com.teamtreehouse;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
System.out.println("Please enter your story, every word should be in _noun/adjective/etc_ format.");
Prompter prompter = new Prompter();
String story = null;
try {
story = prompter.promptForStory();
} catch (IOException ioe) {
ioe.getMessage();
ioe.printStackTrace();
}
}
}
This is the most recent Prompter.java file:
package com.teamtreehouse;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
System.out.println(tmpl.render(results));
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
/**
* Prompts the user for the answer to the fill in the blank. Value is guaranteed to be not in the censored words list.
*
* @param phrase The word that the user should be prompted. eg: adjective, proper noun, name
* @return What the user responded
*/
public String promptForWord(String phrase) throws IOException {
System.out.println("Please enter a " + phrase);
String enteredWord = mReader.readLine();
while (mCensoredWords.contains(enteredWord.toLowerCase())) {
System.out.printf("%s is not appropriate.", enteredWord);
System.out.println("Please try again entering a proper " + phrase + " and continue with your story telling.");
enteredWord = mReader.readLine();
}
return enteredWord;
}
public String promptForStory() throws IOException {
//prompt a user for a story
Prompter prompter = new Prompter();
String story = mReader.readLine();
Template tmpl = new Template(story);
prompter.run(tmpl);
return story;
}
}
Every time when I check work after pasting my code into challenge, it says:
java.lang.NullPointerException ( look around Prompter.java line 80 )
that is:
while (mCensoredWords.contains(enteredWord.toLowerCase())) {
( I have closed this while statement with the right curly bracket "}" after the section of code in this while statement in the Prompter.java file )
I click preview, This is what displays:
Please enter your story, every word should be in _noun/adjective/etc_ format.
Please enter a first prompt
java.lang.NullPointerException
at com.teamtreehouse.Prompter.promptForWord(Prompter.java:80)
at com.teamtreehouse.Prompter.promptForWords(Prompter.java:62)
at com.teamtreehouse.Prompter.run(Prompter.java:40)
at com.teamtreehouse.Prompter.promptForStory(Prompter.java:94)
at com.teamtreehouse.Main.main(Main.java:16)
at JavaTester.run(JavaTester.java:77)
at JavaTester.main(JavaTester.java:39)
Before I copy and paste the code into challenge, I run it in intelliJ, it says:
Please enter your story, every word should be in _noun/adjective/etc_ format.
Couldn't load censored words
java.nio.file.NoSuchFileException: resources/censored_words.txt
.
.
.
process finished with exit code 1.
Help is needed. I still can not pass this challenge. My understanding is we ask a user to enter their story, we compare each word in their story to the words in the censored_words.txt to see if it matches any censored word; if it does, we tell the user the word is not appropriate and ask the user to try again and enter the proper phrase and continue finish telling his/her story.
3 Answers
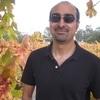
Kourosh Raeen
23,733 PointsI see a problem in your Main.java class. you have this line:
Template tmpl = new Template(story);
But at this point story is null. Move this line after the try catch block. Also after creating the Template pass it to the run method of the Prompter class:
package com.teamtreehouse;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
Prompter prompter = new Prompter();
String story = null;
try {
story = prompter.promptForStory();
} catch (IOException e) {
e.printStackTrace();
}
Template tmpl = new Template(story);
prompter.run(tmpl);
}
}

Peng Liu
Courses Plus Student 14,273 Pointshi, everyone, I see a censored_words.txt in resources folder, there are four censored words in it. Based on my most code, it gives me error message says "could't load censored words" that is the error message displayed after caught by catch block. The line of code in the try block "words = Files.readAllLines(file);" did not read all those censored words in file(refers to censored_words.txt from my understanding), that is why catch block error message displayed that message about could't loading censored words. I need to fix "words = Files.readAllLines(file)", so the catch block will not catch any error message; the flow of code go directly to "mCensoredWords.addAll(words)", I need to write something to the right side of "words = something", "readAllLines(file)" does not read those censored words currently, that is why consequently it does not load those censored words. This is just my opinion, it could be wrong.

Craig Dennis
Treehouse TeacherHi Peng Liu !
Did you get this figured out? There should be a resources folder at the same root as your src file with a file called censored_words.txt. Does it exist there?

Peng Liu
Courses Plus Student 14,273 PointsHi, Craig:
I send a email to you, mentioned that "words = Files.readAllLines(file);" which file refers to that censored_words.txt file in resources folder, is the line bothered me, it could be the reason when I debug, it always says "could not load the censored words." Oh, those text mixed in loadCensoredWords() code block says "e: No suchFileException..." or any other words in that section of code in my email is just message get from my IDE after I debug and step into each step of that block of code. Please use code of my most updated one here as reference, thanks!

Craig Dennis
Treehouse TeacherIs there a file in your resources folder called censored_words.txt ? Is there information in it? In the original download it is there. Do you see it?

Craig Dennis
Treehouse TeacherWeird!?
I haven't seen this problem before...if you can see the file, that code should be able to read it. Can you zip up your directory and email it to me please? craig at teamtreehouse.com
Thanks!
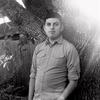
Oziel Perez
61,321 PointsIn case you still haven't figured it out, the resources folder should be all the way at the root directory. In this case, it would be the TreeStory folder, which contains the src folder, the .idea folder, and the iml file. Your resources folder should be sitting next to these other things, with the txt file inside of it. I had a similar problem; I knew that I was getting this error because the folder wasn't in the correct place but I just didn't know what place that was. You just have to remember that Paths is going to start searching all the way at the top of your working directory, which will be TreeStory folder.

Peng Liu
Courses Plus Student 14,273 PointsHi, Oziel:
Thank you for your comment, I have double checked my files and directory, the resource folder (with censoredwords.txt file in it) and everything else are in the right places. It still does not compile successfully, could not find the censored words.txt file. I have stepped into and through that part of codes, right after Files.readAllLines() method seams could not find the censored words.txt, that could be the reason it catches exception error and displayed the message "could not load the censored words.". Anyway, thank you for your time on this issue and my question, sorry for the whole coding section, it takes time to read, I feel that is necessary and easier for people to communicate with each other.
Appreciated! Peng
Peng Liu
Courses Plus Student 14,273 PointsPeng Liu
Courses Plus Student 14,273 PointsHi, everyone, this is my updated Main.java file: