Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial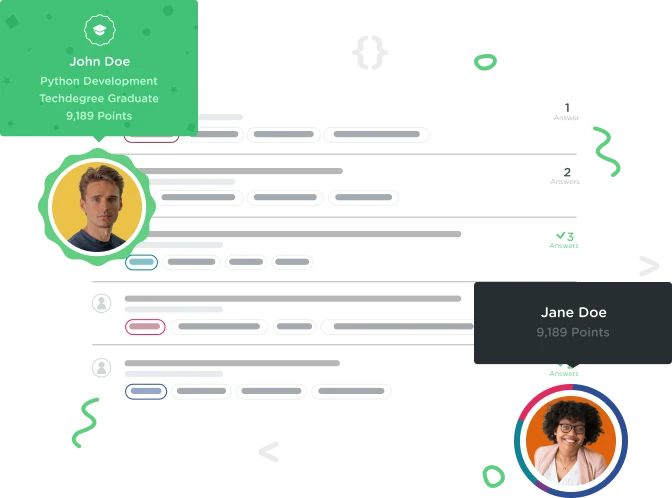
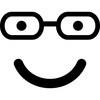
prateekmahesh
5,454 PointsCan I have the solution?
Can someone help me with this task?
def disemvowel(word):
for i in word:
if i == "a" or i == "A" or i == "e" or i == "E" or i == "i" or i == "I" or i == "o" or i == "O" or i == "u" or i == "U":
word.remove(i)
return word
2 Answers
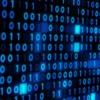
Alexander Davison
65,469 PointsYou can't call remove
on a string, but you can do it on lists.
Try making a new variable called result
(you can call it whatever you want; I like it to be called result
) and use it to store the value of word
in a list form. To convert strings to lists, you can use the list
function (as shown in Figure 1). Then, replace all usages of word
after this line of code with result
(or whatever you called it).
Figure 1:
>>> list('abc')
['a', 'b', 'c']
>>> list('prateek is cool')
['p', 'r', 'a', 't', 'e', 'e', 'k', ' ', 'i', 's', ' ', 'c', 'o', 'o', 'l']
I hope this helps.
If you need further help, please reply. I'd be happy to help furthermore.
~Alex

Samuel Focht
4,321 Pointsdef disemvowel(word):
new_word = ""
for letter in word:
if letter not in 'aeiouAEIOU':
new_word += letter
return new_word
Ok what I did was iterate over our variable word. Now important!!!!!! You can't change a string. So I made a new variable. I tested each letter to see if it was a vowel if it wasn't (not in) it was added to new_word. Hope this helped.