Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial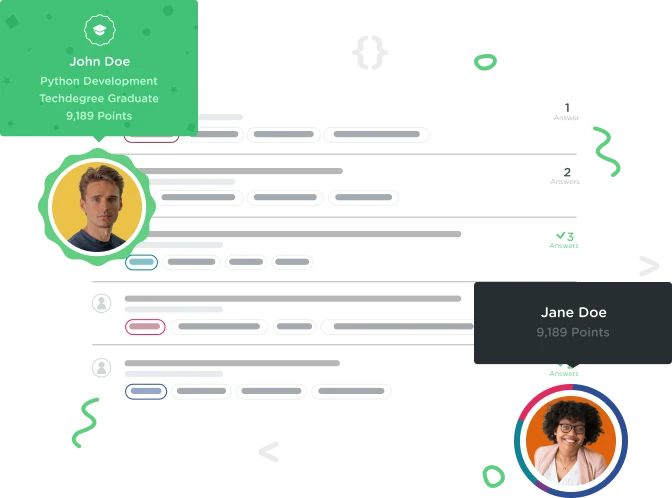

Abby Holzinger
1,293 PointsCall Method
How do I use the getAuthor() method to work in the Blog class from the BlogPost class? And if I can't do that, how do I get the for loop to go through all the blogPosts?
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> results = new TreeSet<String>();
for(String author : getAuthor()) {
results.add(author);
}
return results;
}
}
4 Answers

Iain Diamond
29,379 PointsAbby wrote:
public Set getAllAuthors() {
Set results = new TreeSet();
for(String author : post.getAuthor()) {
results.add(author);
}
return results;
}
Getting the authors happens in two stages. You know that a post has an author, and you have a list of posts, mPosts. So by looping over the posts you can find the authors.
for (BlogPost post: mPosts) {
author = post.getAuthor();
}
Obviously, you want to store these names somewhere:
Set<String> authors = new TreeSet<String>();
Using a TreeSet, keeps the names in alphabetical order. Thus, putting it all together you have:
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<String>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
Remembering to add imports as well.

Iain Diamond
29,379 PointsHi Abby,
The Blog class has an attribute, mPosts, which is a list of posts. You should be able to use a for loop to go through each post and call its getAuthor() method from the BlogPost class, storing each one in your results set.
Hope this helps, iain

Abby Holzinger
1,293 Pointsso should
for(String author : mPosts.getAuthor())
work? because I've tried pretty much every combination there. Unless I need to import something else??

Iain Diamond
29,379 PointsIf you loop over a list of Strings, myStringList, you would write:
for (String s: MyStringList) {
System.out.println(s);
}
So if you have a list of posts, mPosts, you could write:
for (BlogPost post: mPosts) {
System.out.println(post);
}
As the BlogPost class has a method getAuthor() you could use that to get all the author names.
post.getAuthor();
And store the result in your results set.
Hope this helps.

Abby Holzinger
1,293 PointsI've been trying the post.getAuthor() in Blog.java. I even imported com.Example.BlogPost, but when I put this in
public Set<String> getAllAuthors() {
Set<String> results = new TreeSet<String>();
for(String author : post.getAuthor()) {
results.add(author);
}
return results;
}
I still get this error.
./com/example/Blog.java:22: error: cannot find symbol for(String author : post.getAuthor()) { ^ symbol: variable post location: class Blog Note: JavaTester.java uses unchecked or unsafe operations. Note: Recompile with -Xlint:unchecked for details. 1 error
Abby Holzinger
1,293 PointsAbby Holzinger
1,293 Pointsyes! Thank you!