Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial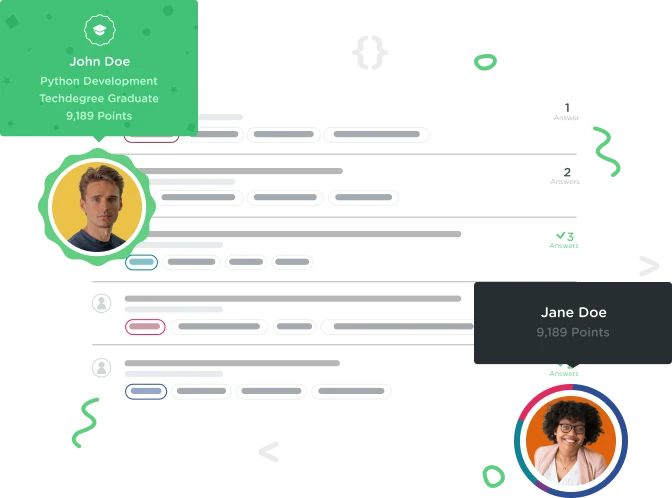
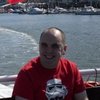
Sean Flanagan
33,235 PointsCall Centre Queue - Task 1
Hi. I included peek() in my code but it's not passing. Can anyone point me towards the correct movie for this exercise please?
Thanks.
import java.util.ArrayDeque;
import java.util.Queue;
public class CallCenter {
Queue<CustomerSupportRep> mSupportReps;
public CallCenter(Queue<CustomerSupportRep> queue) {
mSupportReps = queue;
}
public void acceptCustomer(Customer customer) {
CustomerSupportRep csr;
/********************************************
* TODO (1)
* Wait until there is an available rep in the queue.
* While there is not one available, playHoldMusic
* HINT: That while assignmentcheck loop syntax we used to
* read files seems pretty similar
********************************************
*/
while(mSupportReps.peek() == null) {
playHoldMusic();
}
/********************************************
* TODO (2)
* After we have assigned the rep, call the
* assist method and pass in the customer
********************************************
*/
/********************************************
* TODO (3)
* Since the customer support rep is done with
* assisting, put them back into the queue.
********************************************
*/
}
public void playHoldMusic() {
System.out.println("Smooooooth Operator.....");
}
}
import java.util.List;
import java.util.ArrayList;
public class CustomerSupportRep {
private String mName;
private List<Customer> mAssistedCustomers;
public CustomerSupportRep(String name) {
mName = name;
mAssistedCustomers = new ArrayList<Customer>();
}
public void assist(Customer customer) {
System.out.printf("Hello %s, my name is %s, how can I assist you.%n",
customer.getName(),
mName);
System.out.println("...");
System.out.println("Is there anything else I can help you with?");
mAssistedCustomers.add(customer);
}
public List<Customer> getAssistedCustomers() {
return mAssistedCustomers;
}
}
public class Customer {
private String mName;
public Customer(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
5 Answers
Christopher Augg
21,223 PointsSean,
You had the following in your previous code: (I commented what to keep and what to remove)
while(mSupportReps.peek() == null) {
playHoldMusic();
}
csr = mSupportReps.poll(); //KEEP THIS HERE
/********************************************
* TODO (2)
* After we have assigned the rep, call the
* assist method and pass in the customer
********************************************
*/
csr.assist(customer); //compiler error here
mSupportReps.poll(); //REMOVE THIS ONE
Notice that you are assigning car =mSupportReps.poll(); You need to keep that one.
When I said to remove the extra mSupportReps.poll(); I was referring only to the one after csr.assist(customer);
Regards,
Chris
Christopher Augg
21,223 PointsSean,
Your while loop is fine to complete Todo 1. However, when implementing it this way, you will also need to assign what is in the queue to csr once your while loop breaks. But that can be taken care of for Todo 2. You are not passing this challenge because Todo's 2 & 3 are not completed.
The video covering queues is called Queueing. In this video, Craig introduces how to use documentation by exploring the Queue interface at 1:46. He goes on to show how there are many methods one can use like the add() and poll() methods. While he does not point out peek(), it is just another method that can be used to see if a queue has something available.
The video you are probably asking about is for the Hint provided:
- HINT: That while assignmentcheck loop syntax we used to read files seems pretty similar.
That video is called Custom-Serialization where Craig is showing one way to use syntax for reading a file at 4:50. You can definitely follow along and write the loop for the queue using poll() this way:
while((csr = mSupportReps.poll()) == null) {
playHoldMusic();
}
This will continuously assign null to csr causing the comparison to succeed until something is added to the queue. The difference between your code and this code is that you have to do the assignment separately after the while loop with your code. Therefore you would need to use :
while(mSupportReps.peek() == null) {
playHoldMusic();
}
csr = mSupportReps.poll();
Like I said earlier, your loop is fine as written. It is just another way to implement it. The documentation also shows that the isEmpty() method has been inherited. Therefore, you could also use:
while(mSupportReps.isEmpty()) {
playHoldMusic();
}
csr = mSupportReps.poll();
You can use any of these ways to solve the challenge once you complete the Todos. Now all you need to do is call csr.assist method and pass in customer before putting the csr back into the queue.
I hope this helps.
Regards,
Chris
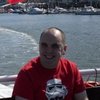
Sean Flanagan
33,235 PointsHi Chris. Thank you for your help. I thought of the to-do's as tasks - my mistake. I realise now that Task 1 is made up of 3 to-do's.
I've given it another go and here's what I've come up with:
import java.util.ArrayDeque;
import java.util.Queue;
public class CallCenter {
Queue<CustomerSupportRep> mSupportReps;
public CallCenter(Queue<CustomerSupportRep> queue) {
mSupportReps = queue;
}
public void acceptCustomer(Customer customer) {
CustomerSupportRep csr;
/********************************************
* TODO (1)
* Wait until there is an available rep in the queue.
* While there is not one available, playHoldMusic
* HINT: That while assignmentcheck loop syntax we used to
* read files seems pretty similar
********************************************
*/
while(mSupportReps.peek() == null) {
playHoldMusic();
}
csr = mSupportReps.poll();
/********************************************
* TODO (2)
* After we have assigned the rep, call the
* assist method and pass in the customer
********************************************
*/
csr.assist(customer); //compiler error here
mSupportReps.poll();
/********************************************
* TODO (3)
* Since the customer support rep is done with
* assisting, put them back into the queue.
********************************************
*/
mSupportReps.add(csr);
}
public void playHoldMusic() {
System.out.println("Smooooooth Operator.....");
}
}
Compiler error:
./CallCenter.java:33: error: variable csr might not have been initialized
csr.assist(customer);
^
Note: ./MockyQueue.java uses unchecked or unsafe operations.
Note: Recompile with -Xlint:unchecked for details.
1 error
Christopher Augg
21,223 PointsSean,
You got it! Just remove the extra mSupportReps.poll(); that you have just after csr.assist(customer);
Regards,
Chris
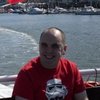
Sean Flanagan
33,235 PointsThanks again Chris.
There's still a compiler error on csr.assist(customer); Am I still doing something wrong?
import java.util.ArrayDeque;
import java.util.Queue;
public class CallCenter {
Queue<CustomerSupportRep> mSupportReps;
public CallCenter(Queue<CustomerSupportRep> queue) {
mSupportReps = queue;
}
public void acceptCustomer(Customer customer) {
CustomerSupportRep csr;
/********************************************
* TODO (1)
* Wait until there is an available rep in the queue.
* While there is not one available, playHoldMusic
* HINT: That while assignmentcheck loop syntax we used to
* read files seems pretty similar
********************************************
*/
while(mSupportReps.peek() == null) {
playHoldMusic();
}
/********************************************
* TODO (2)
* After we have assigned the rep, call the
* assist method and pass in the customer
********************************************
*/
csr.assist(customer); //compiler error reported here
/********************************************
* TODO (3)
* Since the customer support rep is done with
* assisting, put them back into the queue.
********************************************
*/
mSupportReps.add(csr);
}
public void playHoldMusic() {
System.out.println("Smooooooth Operator.....");
}
}
Thanks :-)
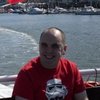
Sean Flanagan
33,235 PointsChris,
You're a lifesaver!!!
Thank you. :-D