Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial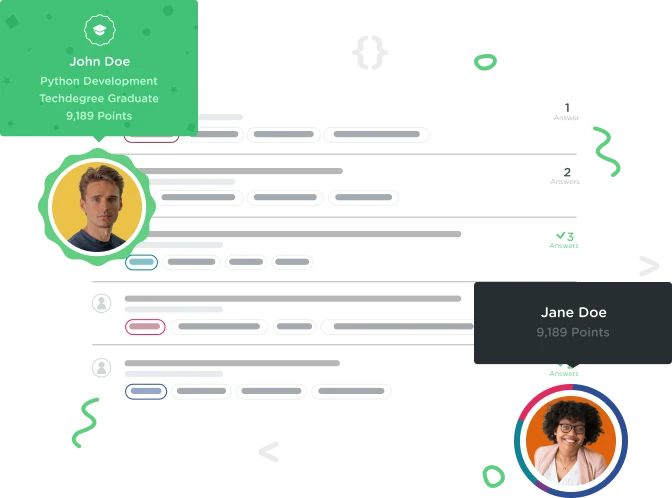
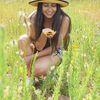
Monica Delgado
2,126 Points"Bummer: It looks like your getter method is not returning a value"
My code is not working, can someone please help me out
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level () {
if (this.credits > 90) {
return 'Senior';
} else if ( this.credits <= 90 && this.credits <= 61) {
return 'Junior';
} else if (this.credits <= 60 && this.credits >= 31) {
return 'Sophomore';
} else if (this.credits <= 30) {
return 'Freshman';
}
}
}
const student = new Student(3.9);
3 Answers
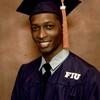
Dane Parchment
Treehouse Moderator 11,077 PointsI'm going to give an in-depth response below. If it's to convoluted for you (sometimes I don't get my thoughts down properly) then simply read the bolded statement for the answer. Feel free to ask for more clarity if you need it. Have fun coding!
There is a very subtle thing about if else-if chains that can be a bit weird with functions that require a return statement. You see in a general if-statement flow: the if-else chain. There are two total possibilities: If and else, thus if you have a return statement in both the if and the else then the function effectively has a return statement because at least one of them will run.
Visual Example
if(number < 10) {
return true;
} else {
return false;
}
The total possible values being either the number is less than 10 or it isn't. So this will either return true otherwise false.
However, in an if-elseif chain, the compiler for javascript can only know whether or not any of the else-if statements are valid at runtime. Understand? In comparison to the above example, the compiler doesn't have just two possible values. So it doesn't read as having a return statement.
To fix this, you must either add an else statement with a return value to your if-else chain, making it an if-else-if-else chain. And thus enforcing at least two values and thus allowing the compiler to determine that your function has a return statement associated to it. Or add a return statement at the end that will run if none of if statements or else if statement pass.
Add either an else statement with a return value in it for when none of the if or else-if statements pass. Or add a return statement at the end of your function that will return if none of the if or else-if statements pass. You also need to check your conditionals to make sure that the proper return values is being used.

jb30
44,807 Points } else if ( this.credits <= 90 && this.credits <= 61) {
should be
} else if ( this.credits <= 90 && this.credits >= 61) {
which can be further simplified to
} else if (this.credits >= 61) {
since only values for this.credit
less than or equal to 90
are left at that point in the code. (If this.credit > 90
was true, your code returned 'Senior' and never got to the else statement.)

Mat T'Qartit
29,277 Pointsour conditional statement is returning the wrong student level.