Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial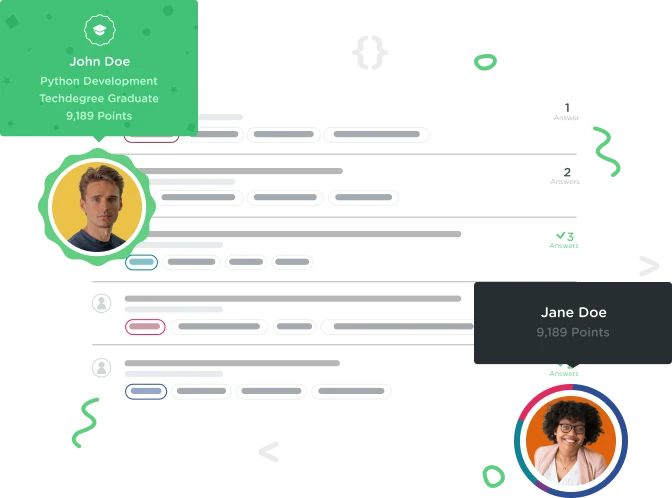
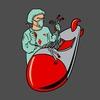
Ryan Dickinson
775 PointsBummer! Hmm, got back letters I wasn't expecting!
def disemvowel(word):
vowels = ['a', 'e', 'i', 'o', 'u']
keep_list = []
for letter in word.lower():
if letter not in vowels:
keep_list.append(letter)
return ''.join(keep_list)
4 Answers

Rogier Nitschelm
iOS Development Techdegree Student 5,461 PointsAs the array has only lowercase letters, it might be a good idea to move the .lower() to the letter inside the if-statement. Because we have to see if the lowercase version of the letter is present in the array (that has just lowercase versions).
Maybe this works?
def disemvowel(word):
vowels = ['a', 'e', 'i', 'o', 'u']
keep_list = []
for letter in word:
if letter.lower() not in vowels:
keep_list.append(letter)
return ''.join(keep_list)
print(disemvowel("I can haz Cheeseburger")) # prints cn hz Chsbrgr

Rogier Nitschelm
iOS Development Techdegree Student 5,461 PointsThe original code turns the entire word to .lowercase before looping over it. However, this will result in the return-value being all-lowercased. This is not desirable because the assignment only requires the vowels to be removed. The capitalization should still be identical.
Right before the moment that you check if a letter is a vowel, you should lowercase it. Which is inside the if-block.
I can imagine this is a little bit confusing, but you could try to put print()-statements inside your function and print the values. This can often clarify what is happening.
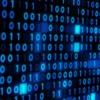
Alexander Davison
65,469 PointsIt doesn't work the first way because in your program you are only returning lowercase letters. I'll show an example.
my_func
is the function that will pass the challenge, and your_func
is the function that WON'T pass the challenge.
Assume I'm in the Python shell
>>> my_func("hello world")
"hll wrld"
>>> your_func("hello world")
"hll wrld"
>>> my_func("HELLO world")
"HLL world"
>>> your_func("HELLO world")
"hll wrld"
>>> my_func("HeLlO WoRlD")
"HLl WRlD"
>>> my_func("Impossible")
"mpssbl"
I hope this clears things up
Happy C0D1NG!
~Alex

Rogier Nitschelm
iOS Development Techdegree Student 5,461 PointsHello there,
Just an observation as I'm not really familiar with python:
It seems you are missing something between the parentheses in the .append()-method. Did you mean to .append(letter)?
Greets, Rogier
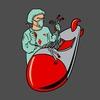
Ryan Dickinson
775 PointsThanks! I have updated the question now. I actually had the argument in there originally, but after reformatting the question post a few times to try and make it look better I had left it out. It's a little wonky to get these questions looking right at first. At least for me. The error message has also been updated.
Ryan Dickinson
775 PointsRyan Dickinson
775 PointsCongrats! You completed the challenge! I don't understand why it doesn't work the original way though. Thanks!