Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial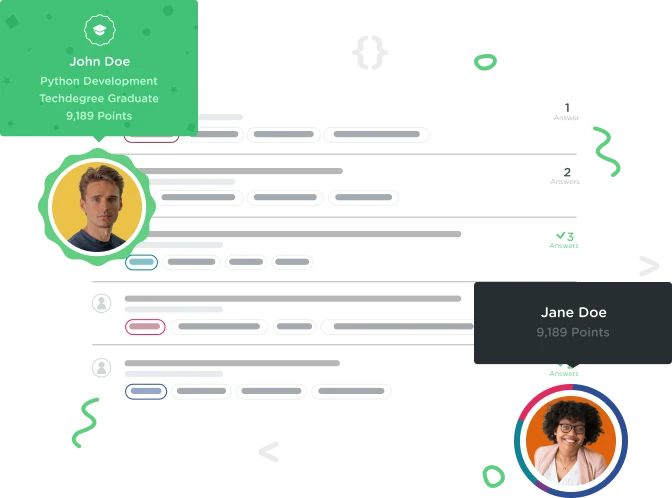

Gokul Nishanth
Courses Plus Student 581 PointsBummer! Didn't get the expected output.
Alright, here's a fun task! Create a function named combiner that takes a single argument, which will be a list made up of strings and numbers. Return a single string that is a combination of all of the strings in the list and then the sum of all of the numbers. For example, with the input ["apple", 5.2, "dog", 8], combiner would return "appledog13.2". Be sure to use isinstance to solve this as I might try to trick you.
Code: def combiner(list1): sum1 = 0 string = "" for i in list1: if isinstance(i, int): sum1 += i elif isinstance(i, str): string += i return "{}{}".format(string, sum1)
def combiner(list1):
sum1 = 0
string = ""
for i in list1:
if isinstance(i, int):
sum1 += i
elif isinstance(i, str):
string += i
return "{}{}".format(string, sum1)
11 Answers
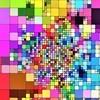
james south
Front End Web Development Techdegree Graduate 33,271 Pointsyou need to tab your return over once to the left to put it under the loop instead of inside it, and then you need to additionally check for floats, not just ints.

Gokul Nishanth
Courses Plus Student 581 PointsThank You.
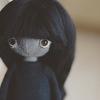
Asma Al-Marrikhi
45,525 Pointsdef combiner(list):
num = 0
str = []
for item in list:
if isinstance(item, int):
num += item
elif isinstance(item, float):
num += item
else:
str.append(item)
return "{}{}".format("".join(str), num)

Christopher Carucci
1,971 PointsSuper simple and clean.
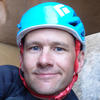
Bob Allan
10,900 Points'str' is a keyword in python, I'm surprised that worked
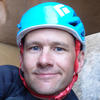
Bob Allan
10,900 PointsA slightly different approach. I hope somebody finds this useful.
def combiner(my_list):
num = 0
s = ""
for el in my_list:
if isinstance(el, str): s += el
else: num += el
return s + str(num);
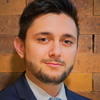
Fernando Suárez
4,772 Pointsdef combiner (list):
words = []
numbers = []
for item in list:
try:
item = float(item)
numbers.append(item)
except ValueError:
if isinstance(item, str):
words.append(item)
numbers = str(sum(numbers))
words = "".join(words)
print(words+numbers)
list = input("Please enter your list values separated by a space ").split( )
combiner(list)
after two hours coming up with this code, I almost killed myself when I saw yours haha... had fun tho

Jake Kobs
9,215 Pointsdef combiner(value):
#Variable to store concatenated strings
Strings = ""
#Variable to store added numbers
Nums = 0
#Loop through the values in value
for val in value:
#Test to see if val is of type float or string
if isinstance(val,(int,float)):
Nums+=val
elif isinstance(val,str):
Strings+=val
#finalValue concatenates the final Strings and Nums variables. Nums is converted to a string value to allow proper concatenation
finalValue = Strings + str(Nums)
return finalValue

nishitbodawala
5,924 Pointsdef combiner(list1): sum1 = 0 string = "" for i in list1: if isinstance(i, int) or isinstance(i, float) : sum1 += i elif isinstance(i, str): string += i return "{}{}".format(string, sum1)
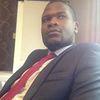
Tapiwanashe Taurayi
15,889 Pointsdef combiner(list): num = 0 str = [] for item in list: if isinstance(item, int): num += item elif isinstance(item, float): num += item else: str.append(item)
return "{}{}".format("".join(str), num)

Stephen Lamm
6,244 PointsAm I the only one that feels entirely unprepared for this challenge? I could have sat here in front of my screen all day and not come up with anything that would have come close to working. Am I alone in this?
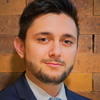
Fernando Suárez
4,772 Pointsyeah, its not just you, its an extremely dificult topic, the first time I got to this point I was completely lost, I skipped OOP and went ahead and continued with the Data Science course. It wasnt untill my third time revisiting the course that I felt like I was actually understanding stuff.

Lazarus Mbofana
10,941 Pointsdef combiner(list): num = 0 str = [] for item in list: if isinstance(item, int): num += item elif isinstance(item, float): num += item else: str.append(item)
return "{}{}".format("".join(str), num)
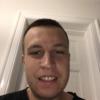
Andrii Gorokh
14,809 PointsWhy this won't work?
def combiner(arg):
result = ""
number = 0
if isinstance(arg, (string, int)) == True:
for i in arg:
if isinstance(i, string) == True:
result.append(i)
else:
number = number+i
final = result+number
return final
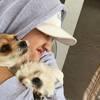
Carlos Marin
8,009 Points1) You should replace string w/ str.
2) if isinstance(arg, (str, int)) == True
equates to False.
why? because the list also contains floating-point values. this problem causes the loop not to run.
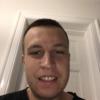
Andrii Gorokh
14,809 PointsThanks1
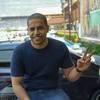
Mohammed Abdelhadi
17,331 Pointsdef combiner(list): num = 0 str = [] for item in list: if isinstance(item, (int,float)): num += item else: str.append(item)
return "{}{}".format("".join(str), num)
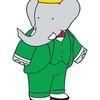
Kitxiba Babar Zakariya
3,117 PointsAny idea why this didn't work?
def combiner(collection):
all_strings = []
all_numbers = 0
for i in collection:
if isinstance(i, str):
all_strings.append(i)
elif isinstance(i, (float, int)):
all_numbers += i
print("{}{}".format(''.join(all_strings), all_numbers))
Kristian Walsh
4,044 PointsKristian Walsh
4,044 PointsThe 'isinstance' for the number also needs to include a float value