Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial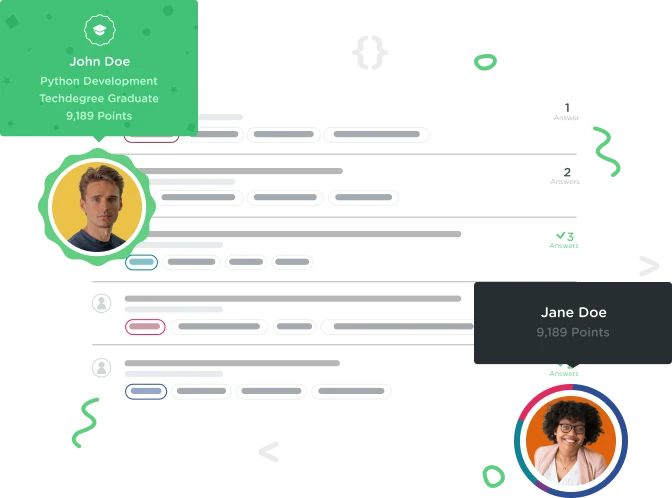

Christopher Bate
4,736 PointsBug in the tester?
I ran this code and the preview shows correctly, however it is constantly saying that task 1 is no longer passing. When I go back it is working fine.
const section = document.querySelector('section');
let paragraphs = section.children;
for (var p in paragraphs){
paragraphs[p].style.color = 'Blue';
}
<!DOCTYPE html>
<html>
<head>
<title>Child Traversal</title>
</head>
<body>
<section>
<p>This is the first paragraph</p>
<p>This is a slightly longer, second paragraph</p>
<p>Shorter, last paragraph</p>
</section>
<footer>
<p>© 2016</p>
</footer>
<script src="app.js"></script>
</body>
</html>
2 Answers

Joel Kraft
Treehouse Guest TeacherHi Christopher,
You are getting this error because paragraphs
is not actually a JavaScript array, but an HTML collection. HTML collections are like arrays, but you cannot depend on them to do everything you could do with an array. This would include for..in
loops, for..of
loops and the forEach
method.
In this case, you're iterating over the collection with a for..in
loop. So the integer properties (0
, 1
, 2
) are being accessed and their style.color
is being set successfully, but when the collection's length
property is accessed in the loop, it doesn't have style.color
, so the challenge is throwing a TypeError behind the scenes. You can see this by pasting your entire code from the second task back into the first task. Admittedly the error you were seeing is not great. We will work on getting a better message to appear for this case.
So as far as working with HTML collections goes, use a for
loop to iterate over them to avoid trouble.

Seth Kroger
56,416 PointsFor whatever reason the challenge only accepts a regular for loop, not a for-in, for-of or forEach().
for (var i=0; i < paragraphs.length; i++){
paragraphs[i].style.color = 'blue';
}