Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial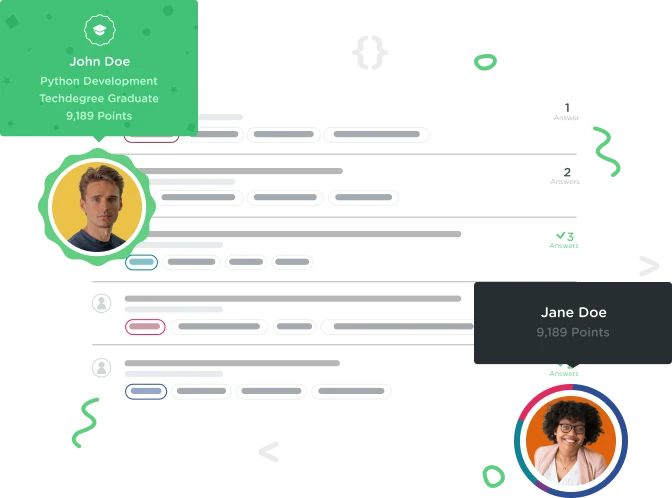
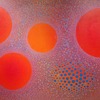
Jef Davis
29,162 PointsBlogPost.getTitle()
I've run into this problem a few times in the past and I've always managed to "fix" it without truly understanding the solution. I've just encountered this error again in trying to complete this activity and the compiler error I get is the following: ./TypeCastChecker.java:18: error: non-static method getTitle() cannot be referenced from a static context result = BlogPost.getTitle(); ^
So, I get what a static and non-static method is, I'm just not sure how it can best be resolved here (and the why of the whole process). Thanks in advance.
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
import com.example.BlogPost;
public class TypeCastChecker {
/***************
I have provided 2 hints for this challenge.
Change `false` to `true` in one line below, then click the "Check work" button to see the hint.
NOTE: You must set all the hints to false to complete the exercise.
****************/
public static boolean HINT_1_ENABLED = false;
public static boolean HINT_2_ENABLED = false;
public static String getTitleFromObject(Object obj) {
String result;
if (obj instanceof String == true) {
result = (String) obj;
}
else if (obj instanceof BlogPost == true) {
result = BlogPost.getTitle();
}
return result;
}
}
2 Answers
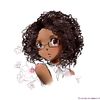
Gloria Dwomoh
13,116 PointsI believe you need to make an instance of the class since your method is non static.
ex.
BlogPost news = new BlogPost();
news.setPost("Yes");
instead of
BlogPost.setPost("Yes");
which would have been okay if your method was static.
I hope this helps.
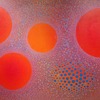
Jef Davis
29,162 PointsOh! Of course, I can't believe I forgot that... it's always the simple things that cause such big problems! Thanks!
Jef Davis
29,162 PointsJef Davis
29,162 PointsThanks for the quick reply. I think you're right that I need to create a new instance of BlogPost here to access the getTitle() method inside a static method here; however, I don't want to create a new instance, but access information from obj passed into the getTitleFromObject method in the TypeCastChecker class. In the first part of the task I completed code in getTitleFromObject to check if obj was a String and return the variable result. The instructions for this part of the exercise are as follows:
Now make sure that if a com.example.BlogPost is passed in for obj that you can cast it to a BlogPost. Return the results of the getTitle method on the newly type-casted BlogPost instance.
I think I need to take obj and downcast as a BlogPost to use the method getTitle, which returns a string.
Jef Davis
29,162 PointsJef Davis
29,162 PointsI tried this:
I get the following error:
./TypeCastChecker.java:21: error: variable result might not have been initialized return result;
So, that line worked in the first half of the exercise when I initialized is on the first line inside the method. Any ideas why initializing the BlogPost title might have caused problems for that?
Gloria Dwomoh
13,116 PointsGloria Dwomoh
13,116 PointsOkay thanks. The problem with that code is "results" variable is not initialized. What happens if the if-else ends up being false in both blocks? Then your return will return what?
From Initial Values of Variables (make sure you read that).
"A local variable (ยง14.4, ยง14.14) must be explicitly given a value before it is used, by either initialization (ยง14.4) or assignment (ยง15.26), in a way that can be verified using the rules for definite assignment (ยง16). "
The editor says
so you should assign in the correct string to that variable and your latest code should be working :)