Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial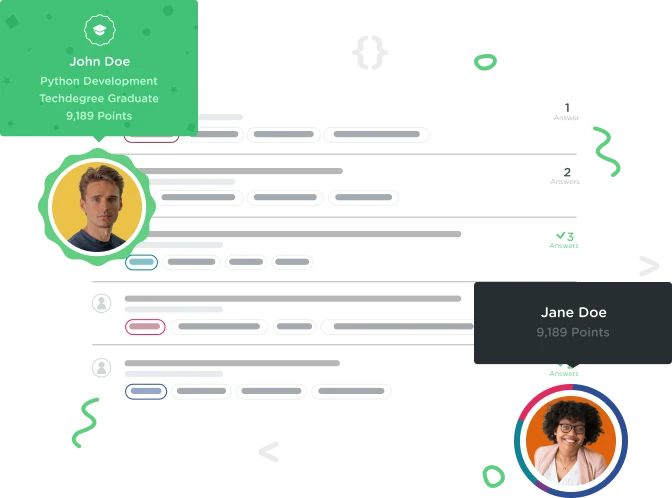

Zimri Leijen
11,835 PointsAvoid repeating multiple `Movie.create()` instructions
I can see if you'd want to add lots of movies you'd get a lot of repeated code.
Doing it this way you don't need to repeat any code and you'll get the exact same results.
(async () => {
await sequelize.sync({ force: true });
try{
const movieCreations = ['Toy Story', 'The Incredibles'].map( title => Movie.create({ title }))
await Promise.all(movieCreations);
} catch(error){
console.error('Error connecting to the database: ', error)
};
}) ();
Edit:
after the end of the course, I ended up with something like this:
(async () => {
await db.sequelize.sync({ force: true });
try {
const movies = [
{title: 'Toy Story', releaseDate: '1995-11-22', runtime: 81, isAvailableOnVHS: true},
{title: 'The Incredibles', runtime: 115, releaseDate: '2004-04-14', isAvailableOnVHS: true},
{releaseDate: '1998-01-15', title: 'Titanic', runtime: 195, isAvailableOnVHS: true},
].map( ({ title, runtime, releaseDate, isAvailableOnVHS } ) => Movie.create({
title,
runtime,
releaseDate,
isAvailableOnVHS
}));
const persons = [
{firstName: 'Leonardo', lastName: 'diCaprio'},
].map( ({ firstName, lastName }) => Person.create({
firstName,
lastName
}));
await Promise.all([...movies, ...persons]);
} catch(error) {
if(error.name === 'SequelizeValidationError') {
const errors = error.errors.map(err => err.message);
console.error('Validation errors:', errors);
} else {
throw error;
}
};
}) ();
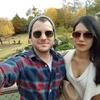
Jamie Gobeille
Full Stack JavaScript Techdegree Graduate 19,573 PointsThat was such a good idea to do it that way! I used this pattern for my project as well!
I separated my arrays and create Functions so it was easier to read(for me at least).
//reusable Function
const mapFunction = (array, model) => {
array.map(
//maps through each object in the array, then destructures it
(object) => model.create({ ...object })
);
};
(async () => {
//Sync all tables
await db.sequelize.sync({ force: true });
try {
//Array of movie data
const movies = [
{
title: "Toy Story",
runtime: 81,
releaseDate: "1995-11-22",
isAvailableOnVHS: true,
},
{
title: "The Incredibles",
runtime: 115,
releaseDate: "2004-04-14",
isAvailableOnVHS: true,
},
];
const people = [
{ firstName: "Tom", lastName: "Hanks" },
{ firstName: "Tim", lastName: "Allen" },
{ firstName: "Brad", lastName: "Bird" },
{ firstName: "Holly", lastName: "Hunter" },
];
// Instance of the Movie class represents a database row
//create movies
const movieCreations = mapFunction(movies, Movie);
//create Person
const peopleCreations = mapFunction(people, Person);
await Promise.all([movieCreations, peopleCreations]);
} catch (error) {
if (error.name === "SequelizeValidationError") {
const errors = error.errors.map((err) => err.message);
console.error("Validation errors: ", errors);
} else {
throw error;
}
}
})();
2 Answers
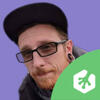
Travis Alstrand
Treehouse TeacherThanks for sharing!
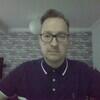
Brian Wright
6,770 PointsSequelize has a Model function bulkCreate(). This works in the same way as create but you pass an array of movie objects instead of a single movie object.
Seokhyun Wie
Full Stack JavaScript Techdegree Graduate 21,606 PointsSeokhyun Wie
Full Stack JavaScript Techdegree Graduate 21,606 PointsI didn't use
...
notation inPromise.all
and it still works perfect. Can anyone explain what are the possible errors or benefits etc. using...
notation particularly in this case? Thanks in advance.