Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial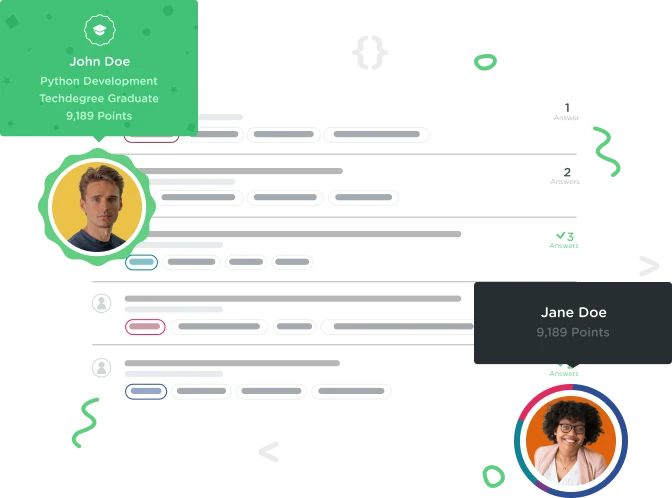
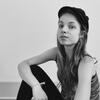
Kate Johnson
Python Development Techdegree Graduate 20,155 PointsArray not defined in password shuffling function
I am trying to write a password function that takes the results of prompts and then converts the string into an array, shuffles the array items, changes the length of the array, joins the array, then converts the array back to a string. I keep receiving the error message in Chrome that my passwordArray is not defined. Could someone please help me? Thank you!
var password = "";
// characters object
var characters = {
lowercase: "abcdefghijklmnopqrstuvwxyz",
uppercase: "ABCDEFGHIJKLMNOPQRSTUVWXYZ",
numeric: "0123456789",
special: "!@#$%^&*()"
};
// Function to generate random number between 8 and 128
var setPasswordLength = function() {
var passwordLength = Math.floor(Math.random()*128)+8;
return passwordLength;
};
// Function to set password characters
var setPasswordCharacters = function() {
// when prompt answered input validated and character type selected
var alphabet, numbers, special;
while (alphabet === undefined) {
var promptCase = window.prompt("Would you like your password to include UPPER case letters? Enter 'YES' or 'NO.'");
switch (promptCase.toLowerCase()) {
case "yes":
alphabet = characters.lowercase + characters.uppercase;
break;
case "no":
alphabet = characters.lowercase;
break;
default:
window.alert("You need to provide a valid answer. Please try again.");
break;
}
}
while (numbers === undefined) {
var promptNumeric = window.prompt("Would you like your password to include numbers? Enter 'YES' or 'NO.'");
switch (promptNumeric.toLowerCase()) {
case "yes":
numbers = characters.numeric
break;
case "no":
numbers = ""
break;
default:
window.alert("You need to provide a valid answer. Please try again.");
break;
}
}
while (special === undefined) {
var promptSpecial = window.prompt("Would you like your password to include special characters? Enter 'YES' or 'NO.'");
switch (promptSpecial.toLowerCase()) {
case "yes":
special = characters.special
break;
case "no":
special = ""
break;
default:
window.alert("You need to provide a valid answer. Please try again.");
break;
}
}
// set password characters based on prompt responses
password = alphabet + numbers + special;
return;
};
// Function to shuffle password characters
var shuffle = function() {
var passwordArray = [];
// convert password to an array
var passwordArray = password.split("");
// randomly sort array items
passwordArray = array.sort(() => Math.random() - 0.5);
// set password length from setPasswordLength()
passwordArray.length = setPasswordLength()
// convert passwordArray back to string
password = passwordArray.join("");
return;
}
// FUNCTION TO GENERATE PASSWORD
var generatePassword = function() {
// prompt and ask for password inputs
setPasswordCharacters();
// shuffle characters in answers to prompts
shuffle();
// password displayed in an alert
window.alert("Your new password is " + password);
};
// Get references to the #generate element
var generateBtn = document.querySelector("#generate");
// Write password to the #password input
function writePassword() {
generatePassword();
var passwordText = document.querySelector("#password");
passwordText.value = password;
}
// Add event listener to generate button
generateBtn.addEventListener("click", writePassword);
2 Answers
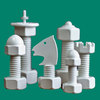
Steven Parker
231,271 PointsI didn't get "passwordArray is not defined", but I did get "array is not defined", referring to this line:
passwordArray = array.sort(() => Math.random() - 0.5);
And, in fact, array
does not appear to be defined anywhere. But since sort affects the array it is called on, what you probably meant to do was this:
passwordArray.sort(a => Math.random() - 0.5);
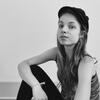
Kate Johnson
Python Development Techdegree Graduate 20,155 PointsThank you! What a silly mistake