Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial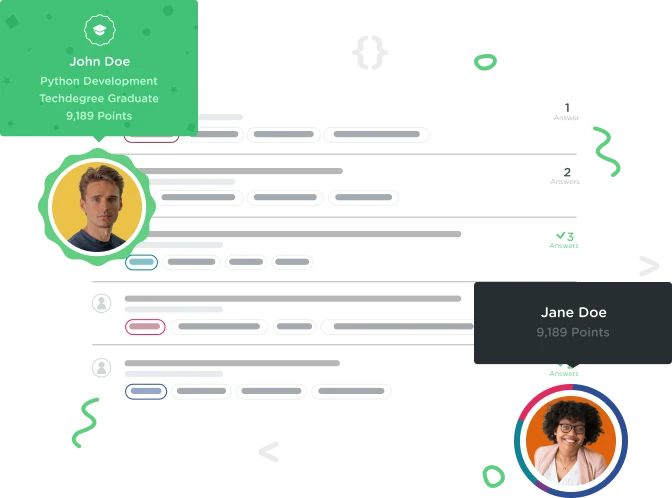
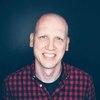
bryonlarrance
16,414 PointsAny suggestions on making this drier and getting it to pass? Thanks!!
Any suggestions on making this drier and getting it to pass? Thanks!!
vowels = ('a','e','i','o','u')
def disemvowel(word):
word_list = list(word.lower())
for letter in word_list:
if letter in vowels:
word_list.remove(letter)
else:
continue
word = str(''.join(word_list))
return word
disemvowel('pirate')
3 Answers
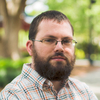
Kenneth Love
Treehouse Guest Teachercontinue
is the default, so you don't need that else
at all. You could just return the ''.join()
bit instead of making it into a variable. You don't technically need the list. You could just assemble a new string from any characters that aren't vowels (and then return that string).
But, as far as passing goes, do the instructions say to lowercase the word?
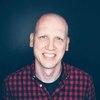
bryonlarrance
16,414 Pointsnew code
vowels = ('a','e','i','o','u','A','E','I','O','U')
def disemvowel(word): word_list = list(word) for letter in word_list: if letter in vowels: word_list.remove(letter) return str(''.join(word_list))
If I remove the word_list how do I get .remove to work without getting an AttributeError?
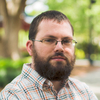
Kenneth Love
Treehouse Guest TeacherYou don't because you can't use .remove()
on a string. If you use a string, instead of a list, you have to do an additive action instead. Make an empty string, then add characters onto it if they're not a vowel.
my_string = 'a1b2'
output = ''
for character in my_string:
if character in '12':
output += character
This is just an example, it isn't a solution to the code challenge :)
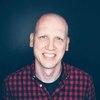
bryonlarrance
16,414 PointsSweet! Thanks Kenneth!
bryonlarrance
16,414 Pointsbryonlarrance
16,414 PointsThanks for the response and for your work with the classes. I am really having a great time!!