Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial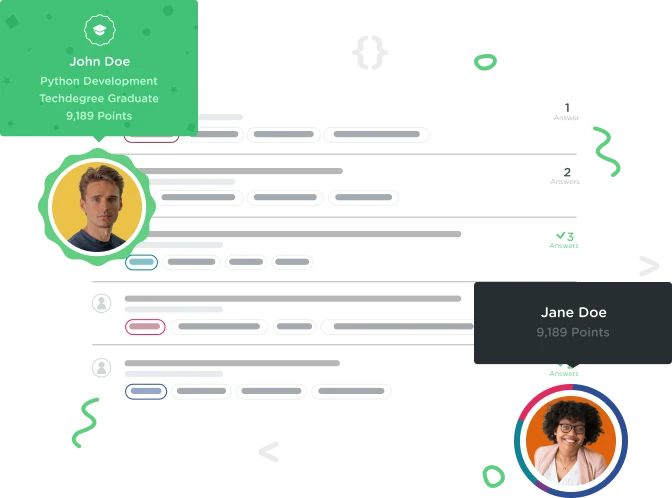

Jimmy Smutek
Python Web Development Techdegree Student 6,629 Points[another] disemvowel problem...
I've been at this for well over an hour and I'm out of ideas. Oddly, and somewhat frustratingly, each of the approaches I take is returning the correct output in my interpreter, but the code challenge is responding with "Bummer! Hmmm, got back letters I wasn't expecting."
Approach 1 - build a new string, based on one of the answers to this thread.
def disemvowel(word):
# list of vowels
vowels = ['a', 'e', 'i', 'o', 'u']
# break word into list, convert to lower case
letters = list(word.lower())
# empty string
disemvoweled = ""
# for each letter
for letter in letters:
# if letter not a vowel
if letter not in vowels:
# add it to new string
disemvoweled += letter
# else continue
else:
continue
# overwrite word
word = disemvoweled
# return
return word
In this case, running print(disemvowel('The quick brown fox jumped over 7 lazy dogs.'))
returns th qck brwn fx jmpd vr 7 lzy dgs.
- which I believe is the correct output?
Alternately, this approach fails with the same error -
def disemvowel(word):
#list of vowels
vowels = ['a', 'e', 'i', 'o', 'u']
# list of letters to iterate through
letters = list(word.lower())
# copy of list to modify
# @see http://stackoverflow.com/a/4960990
disemvoweled = list(word.lower())
# iterate over the letters
for letter in letters:
# if this letter is a vowel
if letter in vowels:
# remove it from the list
disemvoweled.remove(letter)
# overwrite the word variable with new string
word = ''.join(disemvoweled)
# return output
return word
Again, running print(disemvowel('The quick brown fox jumped over 7 lazy dogs.'))
returns th qck brwn fx jmpd vr 7 lzy dgs.
- which is also completely disemvoweled.
What am I missing?
5 Answers
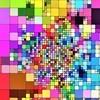
james south
Front End Web Development Techdegree Graduate 33,271 Pointsthe reason is that lowering the whole word lowers the uppercase consonants and it doesn't want that. https://teamtreehouse.com/community/disemvowel-getting-right-answer-but-no-dice

Jimmy Smutek
Python Web Development Techdegree Student 6,629 PointsThis did the trick -
def disemvowel(word):
#list of vowels
vowels = "aeiou"
# list of letters to iterate through
letters = list(word)
# empty string
disemvoweled = ""
# iterate over the letters
for letter in letters:
# if this letter is a vowel
if letter.lower() not in vowels:
# remove it from the list
disemvoweled += letter
# overwrite the word variable with new string
word = disemvoweled
# return output
return word
- First I tried changing the vowels from a list into a string, this didn't help.
- Next I moved the
lower()
method into thefor
loop, as opposed to running it at the same time I made my list of letters, and it passed.
Sooooo it seems that the issue was the placement of the lower()
method. No idea why though.

Jimmy Smutek
Python Web Development Techdegree Student 6,629 PointsThanks James!
Is this Python specific, or do you think this is related to the way Treehouse's system is evaluating the answer?
In this particular scenario it's less efficient to run lower() on the entire word - but it'd be cool to know if this is some obscure (or not so obscure) Python thing to be aware of, or if it's just down to the way the quiz is being evaluated.
Thanks again, I'm marking your answer as best answer since you linked to a related thread.
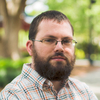
Kenneth Love
Treehouse Guest TeacherIt's not Python-specific or anything obscure or weird like that, it's literally just the instructions to the challenge: remove the vowels. If the instructions don't say to change the case, you shouldn't change the case. Gotta make the software do what the job requires :)

Jimmy Smutek
Python Web Development Techdegree Student 6,629 PointsPerfect, thanks! :)
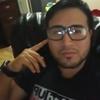
Frank Campos
4,175 Points#this is another way to pass the challenge
def disemvowel(word):
word_for_loop= word
word =''
for letter in word_for_loop:
if letter.lower() in "aeiou":
pass
else:
word= word+letter
return word
disemvowel("abstemious")