Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial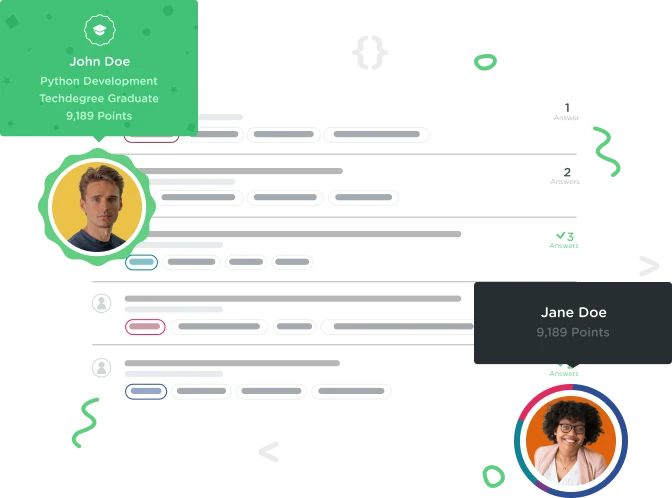
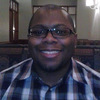
Whitney Barber
9,453 PointsAm I wrong to think the loop will need a function? Is my function broken?
The challenge: For every paragraph element change the color to be blue.
(Hint: remember, paragraphs contains a collection of elements, so you'll need to use a loop and access each element inside.)
My solution below...
const section = document.querySelector('section');
let paragraphs = section.children;
function colorParagraphs (p) {
p.style.color = 'blue';
}
for (i = 0; i < paragraphs.length; i += 1) {
colorParagraphs(paragraphs[i]);
}
<!DOCTYPE html>
<html>
<head>
<title>Child Traversal</title>
</head>
<body>
<section>
<p>This is the first paragraph</p>
<p>This is a slightly longer, second paragraph</p>
<p>Shorter, last paragraph</p>
</section>
<footer>
<p>© 2016</p>
</footer>
<script src="app.js"></script>
</body>
</html>
2 Answers
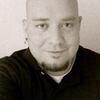
Brian Jensen
Treehouse StaffWell task 1 was to create the variable that captures the children of section => let paragraphs = section.children;
So they are now available via paragraphs. So all you need to do is iterate through paragraphs like so:
for (let i = 0; i < paragraphs.length; i++) {
paragraphs[i].style.color = 'blue';
}
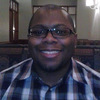
Whitney Barber
9,453 PointsGot it thanks!

Heidi Vasterling-Ford
7,806 PointsWhy is 'let' crucial?

Starky Paulino
Front End Web Development Techdegree Student 6,398 Pointsconst section = document.querySelector('section'); let paragraphs = section.children;
for(let i = 0; i < paragraphs.length; i++) { paragraphs[i].style.color = "blue"; }
Rafael silva
23,877 PointsRafael silva
23,877 Pointsas you see This I will to comment the code to show for you how to work everything...
const section = document.querySelector('section'); // this will read let paragraphs = section.children; // this code will to get all children inside section
for(var i = 0; i < paragraphs.length; i++){ // I have create a for loop paragraphs[i].style.color = 'lightskyblue';
// for you to collect the [i] you need before to type the style you will call first the paragraphs and after the call the [i] loop and after you can add your style like this style.color = 'blue'; }
so I hope help you..