Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial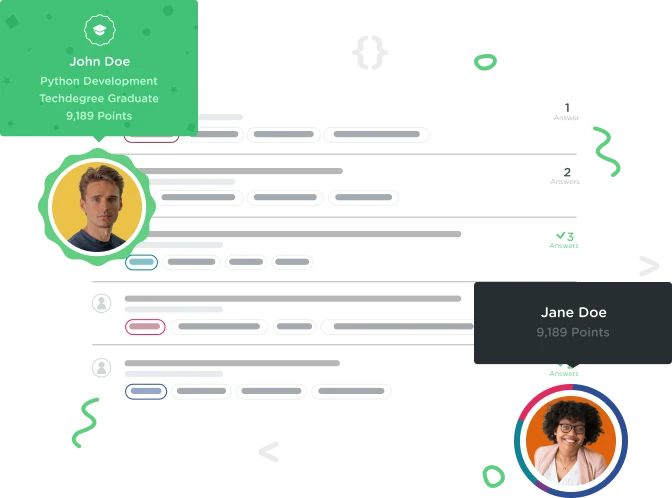

linda wu
3,016 PointsAfter normalizer method is passing, the task one is no longer passing
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
private String normalizeDiscountCode(String discountCode){
for(char code : discountCode.toCharArray()){
if( !(Character.isLetter(code) && code =='$')) {
throw new IllegalArgumentException("Invalid discount code!");
}
}
return this.discountCode=discountCode.toUpperCase();
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
}
5 Answers
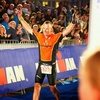
Steve Hunter
57,712 PointsHi Linda,
Two things there. First, you're still trying to assign something in your return
statement. Change:
return discountCode=discountCode.toUpperCase();
to just return the uppercase discount code:
return discountCode.toUpperCase();
Second, you're performing the following test:
if(! Character.isLetter(code) || (code !='$')){
This is using an OR. Let's work through that logic. If we pass in a '$', this is an allowed character so the error shouldn't be thrown. Because this is an OR
, only one side of the ||
needs to evaluate to true
for the exception to be thrown. Character.isLetter('$')
is false
. So, !Character.isLetter('$')
is true
and the exception gets wrongly thrown.
What you're trying to get is a position where a character throws an exception when it is not a letter (you've done that) and it is not a dollar sign. You've not done that comparison; that's not what ||
does.
Let me know how you get on - the issue is with ||
. Try another one!
Steve.
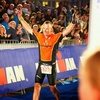
Steve Hunter
57,712 PointsHi Linda,
In your normalizeDiscountCode
method, you just want to return
the value you have normalized. Don't try to assign it to this
as that's what you're doing to the returned value when it arrives back in applyDiscountCode
. So, just return the uppercase version of the discount code that was received as an argument in that method.
Also, I think you need to check the logic in your if
conditional. You're not throwing the error if any character is both a letter and a '$' sign - that's not possible; a letter can't also be a '$'. You're close, but give that area a little more thought.
Let me know how you get on.
Steve.

linda wu
3,016 PointsHi Steve,
I still have problem even after I have updated the code:
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
private String normalizeDiscountCode(String discountCode){
for(char code : discountCode.toCharArray()){
if(! Character.isLetter(code) || (code !='$')){
throw new IllegalArgumentException("Invalid discount code!");
}
}
return discountCode=discountCode.toUpperCase();
}
}

linda wu
3,016 PointsHey Steve,
Thanks for your help. I got it working :))
if(! Character.isLetter(code) && code != '$'){
^^ // error is there!
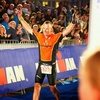
Steve Hunter
57,712 PointsYou got it! Good work.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsP.S. We can trim some brackets too: