Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial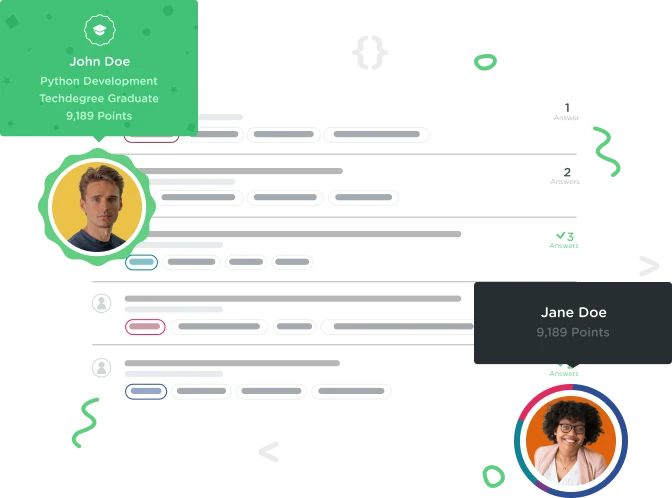
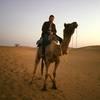
Andrew Warner
4,144 PointsAdding Instant Methods Challenge
Not sure what I am doing wrong here. Can somebody give me a clue on this?
struct Person {
let firstName: String
let lastName: String
func fullName() -> String {
var result = String()
let combined = String("\(firstName) \(lastName)")
result.append(combined)
return result
}
}
1 Answer
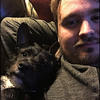
Matthew Long
28,407 PointsYou have the right idea with the string interpolation to create the full name, but you're trying to do too much with the variables result
, combined
, and then trying to append that somewhere. It's much simpler than that. You simply return the interpolated full name string. Often times when it seems like something is over complicated it probably is.
struct Person {
let firstName: String
let lastName: String
func fullName() -> String {
return "\(firstName) \(lastName)"
}
}
The second part of this challenge wants you to create an instance of Person and then use the fullName()
method we created on it. As always, creating an instance of something is done much easier in Xcode.
let aPerson = Person(firstName: "Billy", lastName: "Bobby")
let myFullName = aPerson.fullName()
Hopefully this helps you out and happy coding!