Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial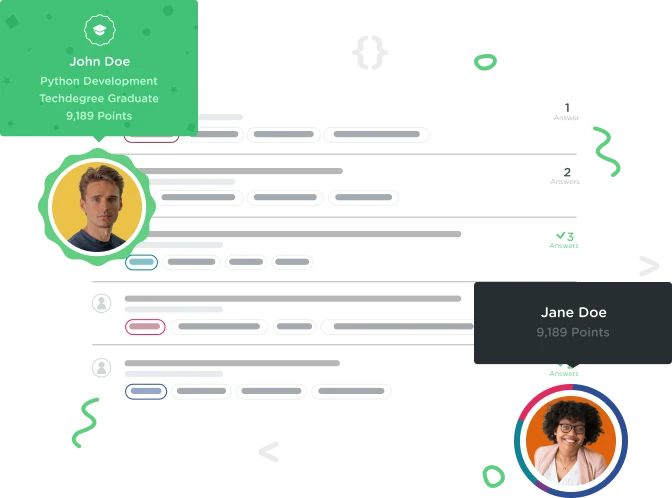
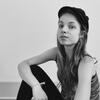
Kate Johnson
Python Development Techdegree Graduate 20,155 Points"addEventListener" null?
Hello, I am trying to create a simple javaScript quiz. When you click the "start quiz" button it should load four other buttons for options a, b, c, and d. However, I am getting this error message in the console "script.js:5 Uncaught TypeError: Cannot read properties of null (reading 'addEventListener') at script.js:5" Does anyone know why?
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Fundamentals Quiz</title>
<link rel="stylesheet" href="./assets/css/style.css">
</head>
<body>
<h1>JavaScript Fundamentals Quiz</h1>
<!-- display questions -->
<div class="quiz-container">
<div id="quiz"></div>
</div>
<!-- buttons -->
<div id="buttons-container" class="buttons-container">
<button id="start">Start Quiz</button>
</div>
<!-- display results -->
<div id="results"></div>
<script src="./assets/js/script.js"></script>
</body>
</html>
var buttonsContainer = document.getElementById("#buttons-container");
var startButton = document.getElementById("#start");
startButton.addEventListener("click", function() {
// create buttonA
var buttonA = document.createElement("button");
buttonA.textContent = "option a";
buttonA.id = "a";
buttonsContainer.removeChild(startButton);
buttonsContainer.appendChild(buttonA);
// create buttonB
var buttonB = document.createElement("button");
buttonB.textContent = "option b";
buttonB.id = "b";
buttonsContainer.appendChild(buttonB);
// create buttonC
var buttonC = document.createElement("button");
buttonC.textContent = "option c";
buttonC.id = "c";
buttonsContainer.appendChild(buttonC);
// create buttonD
var buttonD = document.createElement("button");
buttonD.textContent = "option d";
buttonD.id = "d";
buttonsContainer.appendChild(buttonD);
});
1 Answer
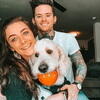
zappbrandigan
21,144 PointsWhen using document.getElementById()
the '#' symbol is not needed. Changing the first two lines of your JS file should fix the error:
var buttonsContainer = document.getElementById("buttons-container");
var startButton = document.getElementById("start");
Kate Johnson
Python Development Techdegree Graduate 20,155 PointsKate Johnson
Python Development Techdegree Graduate 20,155 PointsThank you so much!