Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial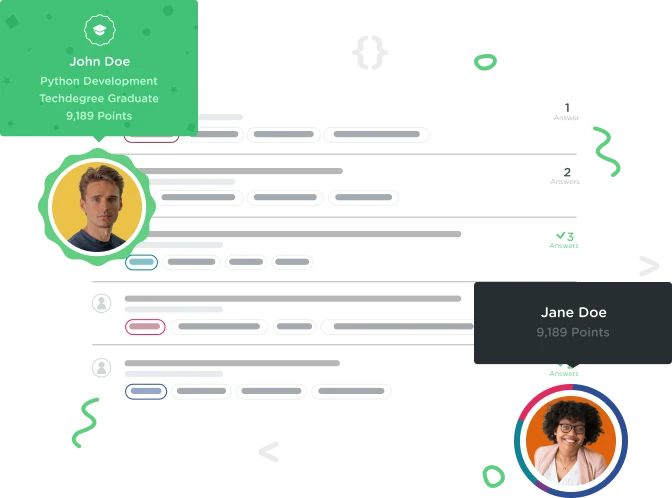

Kevin Gresmer
3,020 Pointsadd a method called getTileCount that uses the for each loop you just learned to loop through the characters and increme
So back to that ScrabblePlayer. I found that it's not enough to know if they just have a tile of a specific character. We need to know how many they actually have. Can you please add a method called getTileCount that uses the for each loop you just learned to loop through the characters and increment a counter if it matches? Return the count, please, thanks!
It keeps telling me i forgot to add a method that accepts a char, but it is telling me to return a count.
Is it not asking for the number of tiles in your hand? Why does it need to accept a char?
Please help.
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount() {
int count = 0;
for (char tile: mHand.toCharArray()) {
if (mHand.indexOf(tile) > -1) {
count++;
}
}
return count;
}
}
2 Answers
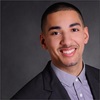
Mario Blokland
19,750 PointsOk, I'll try to take the confusion of your mind a little bit :-)
for (char letter : mHand.toCharArray())
This is saying, hey, I have a String called mHand and I command you to iterate through each character of the String. In each iteration I want only one character at a time and put it in my variable which I named letter. Let's say the String is "test". The method toCharArray() will split the String into an array of characters like this: {'t', 'e', 's', 't'}
.
Now you will iterate through each letter of the char array and can access it as the name letter
.
for (char letter : mHand.toCharArray()) {
System.out.println(letter); // 1st iteration will print 't', 2nd iteration 'e', 3rd iteration 's', 4th iteration 't'
}
Why does your method return 8 with the code you wrote? Well, when is the condition you wrote true?
if (mHand.indexOf(tile) > -1)
If mHand is equal to "test" and tile is equal to 't', then the condition is always true, in each iteration because the letter 't' is always in the String "test". You have to alter the condition to something that only returns true if the value of tile is equal to the current position in the char array. It would look something like this:
- is 't' equal to 't'? If so, count++
- is 't' equal to 'e'? If so, count++
- is 't' equal to 's'? If so, count++
- is 't' equal to 't'? If so, count++
count would hold the value of 2 in this case.
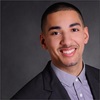
Mario Blokland
19,750 PointsHi Kevin Gresmer,
the method needs to accept a char argument (like the addTile and hasTile method) which you can compare to the string. After that you have to change your if
condition to something that equals true, if the given argument was found in the string. If you use the name tile
as the argument name then don't forget to change the variable name tile
in your for loop otherwise you will have problems with the scope.

Kevin Gresmer
3,020 Pointspublic int getTileCount(char tile) {
int count = 0;
for (char mTile: mHand.toCharArray()) {
if (mHand.indexOf(tile) > -1) {
count++;
}
}
return count;
}
It is now telling me; The hand was "sreclhak" and 'e' was checked. Expected 1 but got 8.
I am really confused. I dont think i fully understand the char mTile: mHand.toCharArray() statement.
Kevin Gresmer
3,020 PointsKevin Gresmer
3,020 PointsThanks for taking the time to break this down. I get it now and passed the challenge.
Mario Blokland
19,750 PointsMario Blokland
19,750 PointsGreat to hear that Kevin! Glad I could help you and awesome that you got it :D
Quinton Rivera
5,177 PointsQuinton Rivera
5,177 PointsCompletely confused partially because of the way the question is worded, Ive spent more than a hour just trying to make sense of the question not i get an error that says The hand was "sreclhak" and 'e' was checked. Expected 1 but got 8.