Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial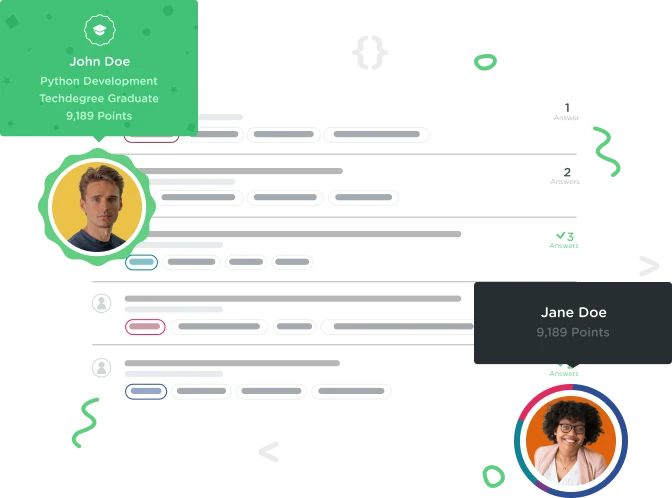

isaac schwartzman
963 PointsAdd a constructor to ForumPost which accepts a User named author, a String named title, and another String named descrip
no idea where I went wrong.
public class Forum {
private String topic;
// TODO: add a constructor that accepts a topic and sets the private field topic
public String getTopic() {
return topic;
}
private String topic = "";
public String getTopic (String topic) {
return topic;
}
public static Topic (String topic){
this.topic = topic;
}
/* Uncomment this when you are prompted to do so
public void addPost(ForumPost post) {
System.out.printf("A new post in %s topic from %s %s about %s is available",
topic,
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle()
);
}
*/
}
public class User {
private String firstName = "";
private String lastName = "";
// TODO: add private fields for firstName and lastName
public static String getFirstName() {
private String firstName = console.readLine("what is your first name?");
return firstName;
}
public static String getLastName(){
private String lastName = console.readLine("what is your last name?");
return lastName;
}
private User(String firstName, String lastName) {
// TODO: set and add the private fields
this.firstName = firstName;
this.lastName = lastName;
}
// TODO: add getters for firstName and lastName
}
public class ForumPost {
private String author = "";
private User author = author;
private String title = "";
private String description = "";
// TODO: add a constructor that accepts the author, title and description
public String ForumPost (User author, String title, String description,) {
this.author = author;
this.title = title;
this.description = description;
return author, title, description;
}
public User getAuthor() {
return author;
}
public String getTitle() {
return title;
}
public String getDescription() {
return description;
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Beginning forum example");
if (args.length < 2) {
System.out.println("Usage: java Main <first name> <last name>");
System.err.println("<first name> and <last name> are required");
System.exit(1);
}
/* Uncomment this when prompted
Forum forum = new Forum("Java");
// TODO: pass in the first name and last name that are in the args parameter
User author = new User();
// TODO: initialize the forum post with the user created above and a title and description of your choice
ForumPost post = new ForumPost();
forum.addPost(post);
// */
}
}
2 Answers

Frank Torchia
18,346 PointsHi Isaac,
Sorry if this is a little wordy. I'm going to go over everything piece by piece.
1 - Forum.java
private String topic = "";
You don't have to assign anything to the topic
variable since the constructor is going to be doing that for us! You can just declare it and leave it unassigned.
public String getTopic (String topic) {
return topic;
}
For your getter I'm seeing you are assigning a parameter to the variable topic
. You would want to remove that since the variable we are "getting" is already contained in the class. This can potentially cause an error when compiling or give you a wrong result all together.
public static Topic (String topic){
this.topic = topic;
}
Someone smarter than me may correct me on this, but I'm pretty sure Java doesn't support static constructors. Instead of public static Topic
just make it public Topic
.
2 - User.java
private String firstName = "";
private String lastName = "";
Same as above. We want to keep the variables unassigned for this exercise since the constructor is doing the assigning.
public static String getFirstName() {
private String firstName = console.readLine("what is your first name?");
return firstName;
}
public static String getLastName(){
private String lastName = console.readLine("what is your last name?");
return lastName;
}
That's awesome that you're going above and beyond of what the exercise is asking you to do by prompting for user input. I have to caution you though because this could cause the exercise to not pass. If you want to build upon the code in the exercise then I would definitely suggest taking it into a Treehouse Workspace. For now though, just remove the lines where you are asking for input.
private User(String firstName, String lastName) {
// TODO: set and add the private fields
this.firstName = firstName;
this.lastName = lastName;
}
Make sure that the constructors you are using are public
instead of private
. There are times when you would actually want a constructor to be private, but it's beyond the scope of this course.
3 - ForumPost.java
private String author = "";
private User author = author;
private String title = "";
private String description = "";
Same as above, leave these unassigned.
public String ForumPost (User author, String title, String description,) {
this.author = author;
this.title = title;
this.description = description;
return author, title, description;
}
Since this is a constructor we aren't actually returning anything. All the constructor does is construct the values by assigning data to them. We will want to remove that return
statement near the bottom.
Hopefully that wasn't too long-winded! Let me know if you have any other questions. I believe that should get you through the 3rd task, which means that there is still the 4th part left to do.
Good luck!

Frank Torchia
18,346 PointsHmm... it might be a couple things.
(User author, String title, String description,)
Try getting rid of the colon after description
Also make sure your constructor is
public ForumPost
Instead of
public String ForumPost
isaac schwartzman
963 Pointsisaac schwartzman
963 PointsTHANK YOU you made a few more things in java click in my head but when I removed return author, title, discreption;i got: Bummer! Make sure you've added a new constructor to the ForumPost class that accepts a User named author, a String named title, and another String named description
any idea what that could be ?