Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial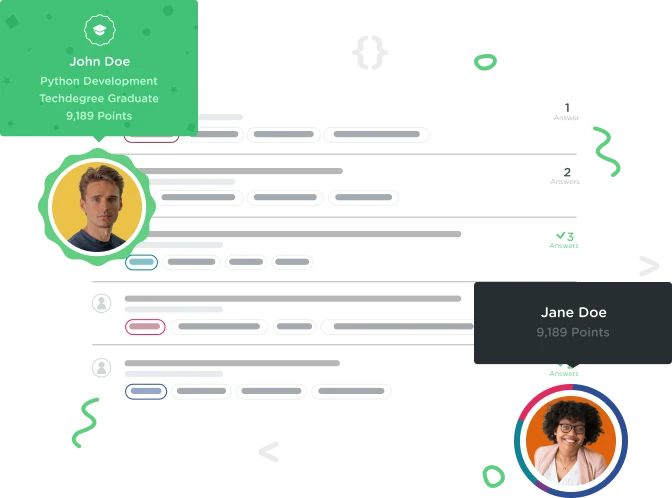

Matt Bloomer
10,608 Points2nd challenge
I don't know what the best way to tackle this challenge; can someone help?
package com.example.model;
import java.util.List;
public class Course {
private String mName;
private List<Video> mVideos;
public Course(String name, List<Video> videos) {
mName = name;
mVideos = videos;
}
public String getName() {
return mName;
}
public List<Video> getVideos() {
return mVideos;
}
}
package com.example.model;
public class Video {
private String mTitle;
public Video(String title) {
mTitle = title;
}
public String getTitle() {
return mTitle;
}
public void setTitle(String title) {
mTitle = title;
}
}
import com.example.model.Course;
import com.example.model.Video;
import java.util.List;
import java.util.Map;
public class QuickFix {
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
List<Video> videoList = course.getVideos();
Video video = new Video("The Beginning Bits");
videoList.add(1, video);
// TODO(2): Add the newly created video to the course videos as the second video.
}
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
public Map<String, Video> videosByTitle(Course course) {
}
return null;
}
}
1 Answer
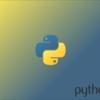
Kevin Faust
15,353 PointsHey Matt,
This was a tricky challenge overall so let's break it down.
So for the first part of the challenge, you did it correctly however there is a shorter way to do it.
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
Video vids= new Video("The Beginning Bits");
// TODO(2): Add the newly created video to the course videos as the second video.
course.getVideos().add(1, vids);
}
Instead of initializing a new list, we can just chain methods. When a Course object is passed through, we can directly call the getVideos() method and then chain it directly to the add() method.
(2) ok for part 2:
For this, we want to loop through the video list in the course object that Craig will pass in. First instantiate a new Map. You can do either a HashMap or TreeMap but it didn't specify in the instructions so either is fine. Using the same method as above (the getVideos() method), we want to get all the videos from the course object. We then loop through that and store each one as a Video object. Then inside the loop, we can now add each video title and oibject to the map. Since maps are unique and dont contain duplicates, we dont have to check whether or not it's already in our map. So get the title of each video and store it as a string. Finally place that title as the fire parameter of the map and the current video we're looping as the second parameter of the map. You could also skip storing the video title as a string and instead just directly put that method inside of the put() method as the first parameter. In case it's still confusing, I put a few solutions below
public Map<String, Video> videosByTitle(Course course) {
Map<String, Video> vidMap = new TreeMap<>();
for (Video currentVids : course.getVideos()) {
String title = currentVids.getTitle(); //store as variable
vidMap.put(title, currentVids); //variable as key, video object as value
}
return vidMap;
}
OR
public Map<String, Video> videosByTitle(Course course) {
Map<String, Video> vidMap = new TreeMap<>();
for (Video currentVids : course.getVideos()) {
vidMap.put(currentVids.getTitle(), currentVids); //doing the above in one step
}
return vidMap;
}
this one is just to show how it would work if it was a list where duplicates are allowed. you wouldnt use this one though in terms of our current scenario
public Map<String, Video> videosByTitle(Course course) {
Map<String, Video> map = new TreeMap<>();
for (Video videos : course.getVideos()) {
if (!map.containsKey(videos.getTitle())) { //if the video title is not in our list then add it otherwise just continue loopin
map.put(videos.getTitle(), videos);
}
}
return map;
}
}
I hope that solved your problems to #2.
If I helped dont forget to mark as best answer so others can see,
Best of luck and happy coding,
Kevin
Dustin Bryce Flanary
17,663 PointsDustin Bryce Flanary
17,663 PointsThanks for the detailed explanation Kevin. I wasn't understanding what Part 2 wanted and that walk-through helped me understand the process and reasoning better.