Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial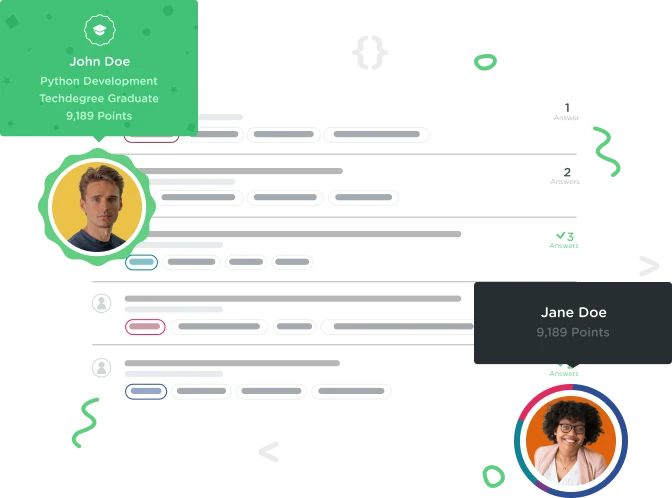

li guopeng
8,873 Pointsupdate a value in a map
I have a problem of update a value in a map, could you please help me passe the last challenge ? we have to update the videotitle.
package com.example.model;
import java.util.List;
public class Course {
private String mName;
private List<Video> mVideos;
public Course(String name, List<Video> videos) {
mName = name;
mVideos = videos;
}
public String getName() {
return mName;
}
public List<Video> getVideos() {
return mVideos;
}
}
package com.example.model;
public class Video {
private String mTitle;
public Video(String title) {
mTitle = title;
}
public String getTitle() {
return mTitle;
}
public void setTitle(String title) {
mTitle = title;
}
}
import com.example.model.Course;
import com.example.model.Video;
import java.util.Map;
import java.util.List;
import java.util.TreeMap;
public class QuickFix {
List<Video> videoList;
Map<String, Video> videoMap;
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
Video videoToAdd = new Video("The Beginning Bits");
// TODO(2): Add the newly created video to the course videos as the second video.
videoList = course.getVideos();
videoList.add(1, videoToAdd);
}
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
Map<String,Video> newvideo = new TreeMap<>();
newvideo = videosByTitle(course);
if(newvideo.containsKey(oldTitle)){
newvideo.put(newTitle,newvideo.get(oldTitle));
newvideo.remove(oldTitle);
}
else{
System.out.println("Title you want to change is not one of the available titles");
}
}
public Map<String, Video> videosByTitle(Course course) {
videoList = course.getVideos();
Map<String, Video> videoMap = new TreeMap<>();
for (Video video : videoList) {
videoMap.put(video.getTitle(), video);
}
return videoMap;
}
}
2 Answers
Christopher Augg
21,223 PointsHello li,
You are very close. Let's see if we can get you on track by going over the last task. This task only require us to complete the fixVideoTitle method by using the videoByTitle method to find the video we need to change. There are different ways to complete this, but we will keep it simple for the purposes of understanding.
We can use a variable to hold a reference of our videosByTitle map by calling the videosByTitle method in which we pass in course. It looks like you did this; however, you made a new map first and then assigned it the reference. It is not necessary to create a new map here. Essentially, what you are doing is assigning a new memory address to a variable of type TreeMap<String, Video> first. When you call the method and assign that to your new variable, you write over that new address with the address of the map that is returned. Therefore, you can just assign the variable with the calling of the method instead of making a new map before hand.
Also, there is no need to use if/else in this case. We just need to retrieve the old video by removing it. We can do that by calling the maps remove method and passing in the oldTitle. Once we have the video, we then use the videos setTitle method to change the name to the new title before using the maps put method to place the video back into the map. Therefore, we don't need the ContainsKey either. I have provided an example of one way this can be completed as an example. You can also use method chaining to get most of this on one line, but that is not the point of this assignment.
Regards,
Chris
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
Map<String, Video> vbt = videosByTitle(course);
Video vid = vbt.remove(oldTitle);
vid.setTitle(newTitle);
vbt.put(newTitle, vid);
}
Christopher Augg
21,223 PointsParson,
No problem. Lets look at the documentation to get a better understanding of what remove does.
The documentation states, "Returns the value to which this map previously associated the key, or null if the map contained no mapping for the key."
Video vid = vbt.remove(oldTitle);
Therefore, when we pass in the Key (i.e. a String) of the old title (i.e oldTitle), the map returns a Value (i.e A Video) because our map is defined as having key/value pairs (String, Video). Once we have a video, we can call its setTitle method to set a new title.
vid.setTitle(newTitle);
And then we can place the video back into the map:
vbt.put(newTitle, vid);
Please let me know if this clears up things for you. I can go into more detail if needed.
Regards,
Chris

Prasoon Shukla
3,426 PointsThanks Chris. The documentation makes it clear. Earlier referred to this http://www.tutorialspoint.com/java/util/hashmap_remove.htm while browsing and got confused. But I understand the difference. If we use remove on a map, it will remove key and value if there is match for the key passed in. After using remove, if we try to print new map, it will show one less pair of key: value. But here we are applying the remove on a map, which inherently returns value for the key being removed from the map.
Prasoon Shukla
3,426 PointsPrasoon Shukla
3,426 PointsHi Chris, Not able to understand, when we use vbt.remove(oldTitle); it should return a map. Why is the value set to Video when Video is not a map? How does .remove work in a map? Does it removes key as well as value for the key? If yes, we should have got a map when we remove a key from the map and not another class (Video in this case).